Python中使用线程池对MySql数据库进行写入操作,数据库写入的操作是通过队列操作还是加锁还是使用事务
时间: 2024-12-12 15:29:33 浏览: 9
在Python中,使用线程池对MySQL数据库进行写入操作通常会结合队列和锁机制来管理和同步数据操作。以下是一个简化的描述:
1. **队列操作**:你可以创建一个任务队列,每个线程从队列中取出写入任务。这种方式可以避免直接访问数据库导致的并发冲突,提高了系统的吞吐量。
```python
from queue import Queue
import threading
# 创建一个任务队列
db_queue = Queue()
def worker_thread():
while True:
task = db_queue.get()
# 执行写入任务到MySQL数据库
write_to_mysql(task)
db_queue.task_done()
# 创建并启动线程池
thread_pool = [threading.Thread(target=worker_thread) for _ in range(thread_num)]
for thread in thread_pool:
thread.start()
# 将写入任务添加到队列
for task in tasks:
db_queue.put(task)
# 等待所有任务完成
db_queue.join()
```
2. **加锁**:为了防止多个线程同时修改数据库,可以在写入操作前获取数据库连接的独占锁。在`write_to_mysql`函数内部使用`with mysql_lock:`来获取锁定,在操作完毕后释放。
```python
import threading
import mysql.connector
mysql_lock = threading.Lock()
def write_to_mysql(task):
with mysql_lock:
cursor.execute(task)
connection.commit()
```
3. **使用事务**:如果你的应用场景需要保证数据的一致性和完整性,那么在每个线程写入之前开启事务,并在完成后提交或回滚事务。这样能确保在异常发生时不会留下部分已完成的数据。
```python
def write_to_mysql(transaction_data):
start_transaction = "START TRANSACTION"
try:
cursor.execute(start_transaction)
# 执行SQL写入操作
...
commit_statement = "COMMIT"
cursor.execute(commit_statement)
except Exception as e:
rollback_statement = "ROLLBACK"
cursor.execute(rollback_statement)
print(f"Error occurred: {e}")
```
阅读全文
相关推荐
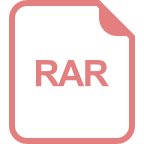
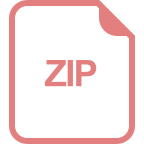
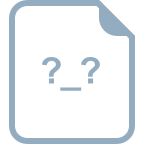
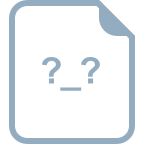
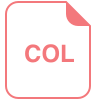
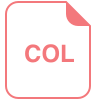
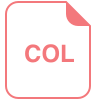
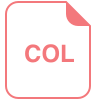
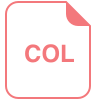
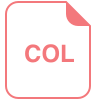
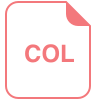
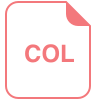
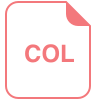
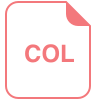
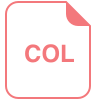
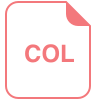
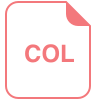
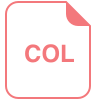
