reverse+sort
时间: 2023-11-12 18:04:44 浏览: 84
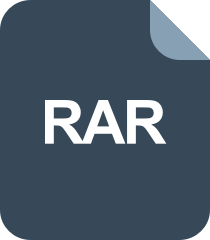
reverse
reverse和sort都是C++ STL中的算法函数,可以用于对容器中的元素进行操作。
reverse函数可以将容器中的元素反转,例如将一个vector从前往后的顺序变为从后往前的顺序。reverse函数的使用方法如下:
```c++
#include <algorithm>
#include <vector>
using namespace std;
int main() {
vector<int> v = {1, 2, 3, 4, 5};
reverse(v.begin(), v.end()); // 将v中的元素反转
return 0;
}
```
sort函数可以对容器中的元素进行排序,例如将一个vector按照从小到大的顺序排序。sort函数的使用方法如下:
```c++
#include <algorithm>
#include <vector>
using namespace std;
int main() {
vector<int> v = {5, 3, 1, 4, 2};
sort(v.begin(), v.end()); // 将v中的元素按照从小到大的顺序排序
return 0;
}
```
如果需要按照从大到小的顺序排序,可以使用sort函数的第三个参数,即自定义比较函数。例如:
```c++
#include <algorithm>
#include <vector>
using namespace std;
bool cmp(int a, int b) {
return a > b; // 按照从大到小的顺序排序
}
int main() {
vector<int> v = {5, 3, 1, 4, 2};
sort(v.begin(), v.end(), cmp); // 将v中的元素按照从大到小的顺序排序
return 0;
}
```
阅读全文
相关推荐
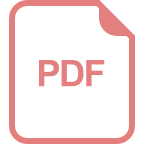
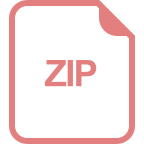
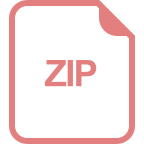
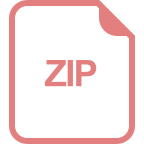
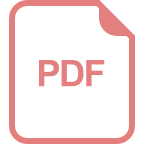




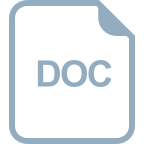






