c++五子棋人人对战
时间: 2023-12-22 09:28:39 浏览: 74
以下是一个简单的C++五子棋人人对战的示例代码:
```c++
#include <iostream>
using namespace std;
const int BOARD_SIZE = 15; // 棋盘大小
// 棋盘类
class Board {
public:
char board[BOARD_SIZE][BOARD_SIZE]; // 棋盘数组
// 构造函数,初始化棋盘
Board() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
board[i][j] = '+';
}
}
}
// 打印棋盘
void printBoard() {
cout << " ";
for (int i = 0; i < BOARD_SIZE; i++) {
cout << i << " ";
}
cout << endl;
for (int i = 0; i < BOARD_SIZE; i++) {
cout << i << " ";
for (int j = 0; j < BOARD_SIZE; j++) {
cout << board[i][j] << " ";
}
cout << endl;
}
}
// 判断是否有五子连珠
bool checkWin(int x, int y, char c) {
int count = 1;
int i, j;
// 横向判断
for (i = x - 1; i >= 0 && board[i][y] == c; i--) {
count++;
}
for (i = x + 1; i < BOARD_SIZE && board[i][y] == c; i++) {
count++;
}
if (count >= 5) {
return true;
}
// 竖向判断
count = 1;
for (j = y - 1; j >= 0 && board[x][j] == c; j--) {
count++;
}
for (j = y + 1; j < BOARD_SIZE && board[x][j] == c; j++) {
count++;
}
if (count >= 5) {
return true;
}
// 左上到右下判断
count = 1;
for (i = x - 1, j = y - 1; i >= 0 && j >= 0 && board[i][j] == c; i--, j--) {
count++;
}
for (i = x + 1, j = y + 1; i < BOARD_SIZE && j < BOARD_SIZE && board[i][j] == c; i++, j++) {
count++;
}
if (count >= 5) {
return true;
}
// 右上到左下判断
count = 1;
for (i = x - 1, j = y + 1; i >= 0 && j < BOARD_SIZE && board[i][j] == c; i--, j++) {
count++;
}
for (i = x + 1, j = y - 1; i < BOARD_SIZE && j >= 0 && board[i][j] == c; i++, j--) {
count++;
}
if (count >= 5) {
return true;
}
return false;
}
};
// 玩家类
class Player {
public:
char color; // 玩家棋子颜色
// 构造函数,设置玩家棋子颜色
Player(char c) {
color = c;
}
// 玩家下棋
void play(Board& board) {
int x, y;
cout << "请玩家 " << color << " 下棋(输入坐标,如 3 4):" << endl;
cin >> x >> y;
while (x < 0 || x >= BOARD_SIZE || y < 0 || y >= BOARD_SIZE || board.board[x][y] != '+') {
cout << "输入有误,请重新输入:" << endl;
cin >> x >> y;
}
board.board[x][y] = color;
}
};
int main() {
Board board; // 棋盘
Player player1('X'); // 玩家1
Player player2('O'); // 玩家2
int turn = 1; // 轮到哪个玩家下棋
while (true) {
board.printBoard();
if (turn == 1) {
player1.play(board);
if (board.checkWin(x, y, player1.color)) {
cout << "玩家 " << player1.color << " 获胜!" << endl;
break;
}
turn = 2;
} else {
player2.play(board);
if (board.checkWin(x, y, player2.color)) {
cout << "玩家 " << player2.color << " 获胜!" << endl;
break;
}
turn = 1;
}
}
return 0;
}
```
阅读全文
相关推荐
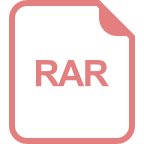
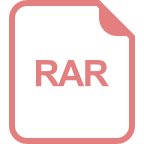
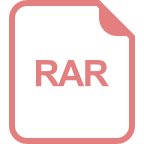
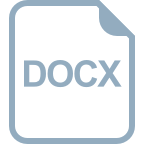
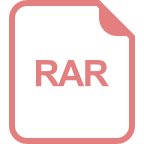
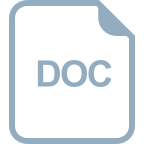
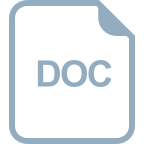






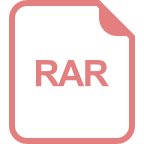
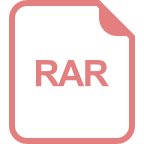