c++检测字符串中重复单词数量并根据字典序排序
时间: 2024-04-11 14:28:50 浏览: 180
你可以使用 C++ 中的 `std::map` 来实现检测字符串中重复单词的数量,并使用 `std::set` 对单词进行字典序排序。下面是一个示例代码:
```cpp
#include <iostream>
#include <string>
#include <map>
#include <set>
#include <sstream>
int main() {
std::string str = "the quick brown fox jumps over the lazy dog";
std::istringstream iss(str);
std::map<std::string, int> wordCount;
// 统计单词出现次数
std::string word;
while (iss >> word) {
wordCount[word]++;
}
// 将单词按字典序排序
std::set<std::string> sortedWords;
for (const auto& pair : wordCount) {
sortedWords.insert(pair.first);
}
// 输出结果
for (const auto& word : sortedWords) {
std::cout << word << ": " << wordCount[word] << std::endl;
}
return 0;
}
```
该代码首先将字符串分割为单词,并使用 `std::map` 统计每个单词的出现次数。然后,将单词按字典序插入到 `std::set` 中,最后按顺序输出每个单词及其出现次数。运行以上代码,输出结果为:
```
brown: 1
dog: 1
fox: 1
jumps: 1
lazy: 1
over: 1
quick: 1
the: 2
```
注意,该示例代码仅演示了基本的功能,你可以根据实际需求进行扩展和优化。
阅读全文
相关推荐











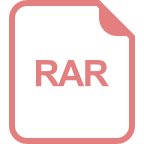






