完成文件中的代码要求
时间: 2024-09-25 19:09:48 浏览: 39
为了帮助您更好地理解和完成“实验3:Pandas 数据分析基础”中提到的任务,我将概述主要步骤以及相应的Python代码示例:
### Pandas基础操作
#### 1. 从列表创建 `Series`
```python
import pandas as pd
s = pd.Series([10, 20, 30, 40, 50], index=['a', 'b', 'c', 'd', 'e'])
print(s)
```
#### 2. 从字典创建 `Series`
```python
s_dict = pd.Series({'a': 1, 'b': 2, 'c': 3, 'd': 4, 'e': 5})
print(s_dict)
```
#### 3. 创建 `DataFrame` 对象
假设我们需要一个包含名字(Name)、年龄(Age)和城市(City)三列的 DataFrame:
```python
data = {'Name':['Tom','Nick','John', 'Jack', 'Lily'],
'Age':[20, 21, 19, 18, 23],
'City':['New York', 'London', 'Singapore', 'Beijing', 'Peking']}
df = pd.DataFrame(data)
print(df)
```
#### 4. 增加数据行与列
- 添加一行:
```python
new_row = {'Name':'Lily', 'Age':23, 'City':'Peking'}
df = df.append(new_row, ignore_index=True)
```
- 在 Age 列之后添加 Sex 列:
```python
df['Sex'] = ['male', 'male', 'male', 'male', 'female']
print(df)
```
#### 5. 选取 ‘Age’ 列并按降序排序
```python
sorted_df = df.sort_values(by='Age', ascending=False)
print(sorted_df)
```
### Pandas统计分析
首先需要构造样例 DataFrame 来模拟题目描述的情况。这里假设有学生的姓名(Name),及他们不同科目(如语文(Chinese)、数学(Mathematics)、英语(English))的成绩。
```python
grades_data = {
"Name": ["Li Hong", "Zhang Ming", "Wang Jiang", "Zhao Li"],
"Chinese": [80, 75, 88, 92],
"Mathematics": [88, 92, 70, 80],
"English": [95, 85, 82, 90]
}
grades_df = pd.DataFrame(grades_data)
```
接下来按照题目要求依次实现各项功能:
#### 计算每位同学各科成绩的平均分
```python
average_scores = grades_df.mean(axis=1)
print("Average scores:\n", average_scores)
```
#### 分别对语文、数学、英语进行分组,计算每组内平均分,并找出每门课的最高/最低分
```python
for subject in ['Chinese', 'Mathematics', 'English']:
print(f"\nSubject: {subject}")
print(grades_df[subject].describe())
```
#### 找到所有数学成绩高于80分的学生的姓名
```python
high_math_students = grades_df[grades_df['Mathematics'] > 80]['Name']
print("\nStudents with Math score over 80:", high_math_students)
```
#### 使用透视表计算每门课程的平均分等信息
```python
pivot_table = grades_df.pivot_table(index='Name', values=['Chinese', 'Mathematics', 'English'], aggfunc={'Chinese': np.mean, 'Mathematics': np.max, 'English': np.min})
print(pivot_table)
```
请注意上述代码仅为示例性质,在实际应用前,请根据具体需求调整数据源及处理逻辑。
阅读全文
相关推荐



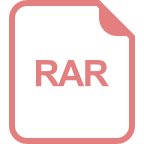



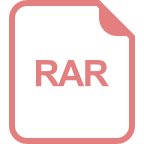




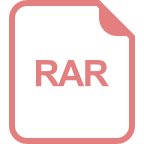
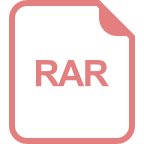
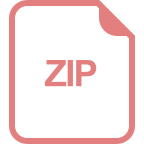

