c语言生产者消费者问题
时间: 2023-08-24 18:21:27 浏览: 136
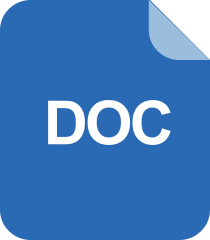
生产者消费者问题C语言
生产者消费者问题是一个经典的并发编程问题,它涉及到两个线程之间的协作,其中一个线程是生产者,负责生成数据,另一个线程是消费者,负责消费数据。在C语言中,可以使用线程库(如pthread)来实现生产者消费者问题的解决方案。
下面是一个基本的C语言生产者消费者问题的解决方案:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define BUFFER_SIZE 10
int buffer[BUFFER_SIZE];
int count = 0;
int in = 0;
int out = 0;
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t full = PTHREAD_COND_INITIALIZER;
pthread_cond_t empty = PTHREAD_COND_INITIALIZER;
void *producer(void *arg) {
int item;
while (1) {
item = rand();
pthread_mutex_lock(&mutex);
if (count == BUFFER_SIZE) {
pthread_cond_wait(&empty, &mutex);
}
buffer[in] = item;
in = (in + 1) % BUFFER_SIZE;
count++;
pthread_mutex_unlock(&mutex);
pthread_cond_signal(&full);
}
return NULL;
}
void *consumer(void *arg) {
int item;
while (1) {
pthread_mutex_lock(&mutex);
if (count == 0) {
pthread_cond_wait(&full, &mutex);
}
item = buffer[out];
out = (out + 1) % BUFFER_SIZE;
count--;
pthread_mutex_unlock(&mutex);
pthread_cond_signal(&empty);
printf("Consumed item: %d\n", item);
}
return NULL;
}
int main(int argc, char *argv[]) {
pthread_t producer_thread, consumer_thread;
pthread_create(&producer_thread, NULL, producer, NULL);
pthread_create(&consumer_thread, NULL, consumer, NULL);
pthread_join(producer_thread, NULL);
pthread_join(consumer_thread, NULL);
return 0;
}
```
在这个例子中,我们使用一个大小为10的循环缓冲区来存储数据,生产者线程随机生成数据并将其添加到缓冲区中,同时消费者线程从缓冲区中消费数据。我们使用互斥锁(mutex)来保护缓冲区,以确保每个线程的操作不会相互干扰。我们还使用条件变量(condition variable)来通知线程何时可以生产或消费数据。当缓冲区已满时,生产者线程将会阻塞等待,当缓冲区为空时,消费者线程将会阻塞等待,直到有新的数据被生产或可消费。
需要注意的是,生产者和消费者线程都是无限循环的,这意味着它们将一直运行直到程序被终止。如果需要退出线程,可以使用pthread_cancel函数来取消线程。
阅读全文
相关推荐
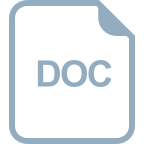
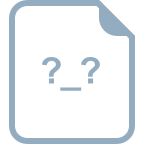
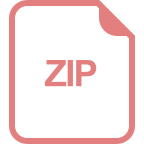


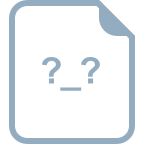
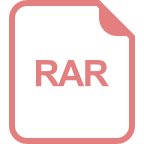
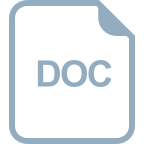





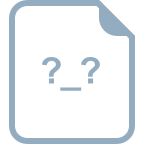