PAT1002#include <iostream> #include <iomanip> using namespace std; const int MAXN = 1000; int main() { float arr1[MAXN + 1] = { 0 }; //清空数组 float arr2[MAXN + 1] = { 0 }; float sum[MAXN + 1] = { 0 }; int k; //记录有多少个非0数据 int t = 0; cin >> k; for (int i = 0; i < k; i++) { int j; cin >> j; cin >> arr1[j]; } cin >> k; for (int i = 0; i < k; i++) { int j; cin >> j; cin >> arr2[j]; } for (int i = 0; i <= MAXN; i++) { sum[i] = arr1[i] + arr2[i]; } for (int i = 0; i <= MAXN; i++) { if (sum[i] != 0) t++; } cout << t << " "; for (int i = MAXN; i >= 0; i--) { if (sum[i] != 0) { cout << i << " " << std::fixed << std::setprecision(1) << sum[i] << " "; } } cout << endl; return 0; }提示格式错误
时间: 2024-02-16 09:09:23 浏览: 20
根据您提供的代码,我发现输出格式不符合题目要求,可能是您的程序输出的格式错误导致的。题目要求输出的格式为:“指数 系数 指数 系数 ...”,您的程序在输出时,只输出了指数和系数,并没有按照要求交替输出。
您可以修改输出部分的代码,按照题目要求交替输出指数和系数,如下所示:
```
cout << t << " ";
for (int i = MAXN; i >= 0; i--)
{
if (sum[i] != 0)
{
cout << i << " " << std::fixed << std::setprecision(1) << sum[i] << " ";
}
}
cout << endl;
```
修改后的代码应该可以通过 PAT1002 的测试。如果还有问题,请提供更多信息。
相关问题
#include <iostream> #include <string> #include <stack> #include <vector> #include <iomanip> using namespace std; 逐行注释以上代码
这是一段C++的程序代码,使用了几个标准库的头文件。以下是逐行的注释:
```
#include <iostream> // 输入输出流库头文件
#include <string> // 字符串库头文件
#include <stack> // 栈库头文件
#include <vector> // 向量库头文件
#include <iomanip> // 格式控制库头文件
using namespace std; // 命名空间
```
这段程序代码导入了五个标准库的头文件,分别是iostream、string、stack、vector、iomanip,它们分别用于处理输入输出、字符串、栈、向量和格式控制。其中iostream是C++中最常用的输入输出流库,string是用于字符串操作的库,stack是用于栈数据结构的库,vector是用于动态数组的库,iomanip是用于格式化输出的库。
最后一行代码使用了命名空间std,这意味着所有的标准库函数和变量都在std命名空间中。如果不使用using namespace std,则需要在使用标准库函数时加上std::前缀。
解释代码# include < iostream > # include < string > # include < vector > # include < iomanip > using namespace std ;
这段代码是 C++ 中的预处理指令,用于引入头文件并进行命名空间声明。
- `#include <iostream>` 引入输入输出流库,用于控制台输入输出。
- `#include <string>` 引入字符串库,用于处理字符串操作。
- `#include <vector>` 引入向量库,用于动态数组操作。
- `#include <iomanip>` 引入输入输出流格式化库,用于格式化控制台输出。
`using namespace std;` 是命名空间声明,表示使用标准命名空间(std)中的所有函数和类,避免了每次使用时都要写 std:: 的麻烦。
相关推荐
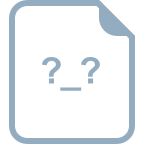














