c语言实现base64编解码
时间: 2024-10-17 20:02:18 浏览: 50
Base64是一种常用的字符编码方式,常用于在网络传输中将二进制数据转换成ASCII字符形式,以便于文本显示。在C语言中,你可以使用标准库函数`openssl/bio.h`或第三方库如`zlib`、`iconv`来实现Base64的编解码。
**编码示例**(使用`openssl`库):
```c
#include <openssl/bio.h>
#include <openssl/evp.h>
void base64_encode(char *input, char *output) {
BIO *bio_in = BIO_new_mem_buf(input, -1);
BIO *bio_out = BIO_new(BIO_f_base64());
bio_out = BIO_push(bio_out, BIO_new_fp(output, "w"));
if (!BIO_write(bio_in, output, strlen(input))) {
// 处理错误
}
BIO_free_all(bio_in);
BIO_free_all(bio_out);
}
```
**解码示例**(同样使用`openssl`库):
```c
#include <openssl/bio.h>
#include <openssl/evp.h>
void base64_decode(const char *encoded, char *decoded) {
BIO *bio_in = BIO_new_mem_buf(encoded, strlen(encoded));
BIO *bio_out = BIO_new(BIO_f_base64());
bio_out = BIO_push(bio_out, BIO_new_fp(decoded, "wb"));
int result = BIO_read(bio_in, decoded, strlen(encoded));
if (result > 0 && result == BIO_get_num_bytes(bio_in)) {
// 解码成功
} else {
// 处理错误
}
BIO_free_all(bio_in);
BIO_free_all(bio_out);
}
```
阅读全文
相关推荐
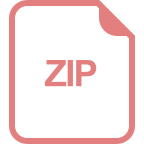
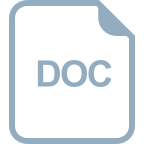
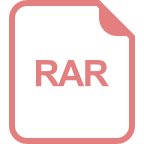
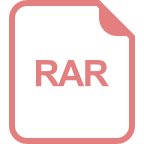
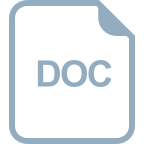
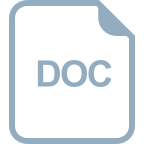
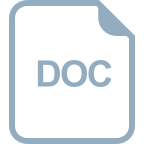

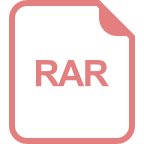
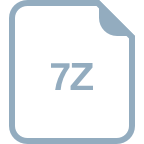
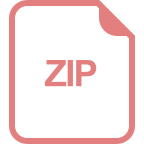

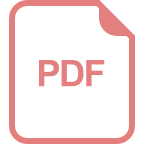