python动态爱心波形代码
时间: 2023-11-17 19:02:13 浏览: 93
Python动态爱心波形代码可以使用matplotlib库来实现。下面是一个简单的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
t = np.arange(0, 2*np.pi, 0.01)
x = 16 * np.sin(t)**3
y = 13 * np.cos(t) - 5 * np.cos(2*t) - 2 * np.cos(3*t) - np.cos(4*t)
line, = ax.plot(x, y)
for i in range(100):
x = 16 * np.sin(t + i/10)**3
y = 13 * np.cos(t + i/10) - 5 * np.cos(2*(t + i/10)) - 2 * np.cos(3*(t + i/10)) - np.cos(4*(t + i/10))
line.set_xdata(x)
line.set_ydata(y)
fig.canvas.draw()
plt.pause(0.01)
plt.show()
```
这段代码使用了numpy和matplotlib库,通过不断改变x和y的值,实现了动态的爱心波形效果。你可以将这段代码复制到Python环境中运行,看看效果。
相关问题
python动态爱心颜色代码
Python 中绘制动态爱心形状并改变颜色通常会涉及到 Pygame 或 Turtle 等图形库。这里以 Python 的 turtle 库为例,展示如何创建一个简单的动态爱心,同时改变其颜色:
```python
import turtle
# 设置画笔
t = turtle.Turtle()
t.speed(0) # 加快绘制速度
def draw_heart(color):
t.color(color)
for _ in range(2): # 画两次心形,一次正向,一次反向
t.begin_fill() # 开始填充颜色
t.penup()
t.goto(-50, -75) # 移动到起点位置
t.pendown()
# 正面心形路径
t.forward(100)
t.right(60)
t.forward(141)
t.right(120)
t.forward(100)
t.right(60)
# 反面心形路径,与正面相反
t.forward(-100)
t.right(-60)
t.forward(-141)
t.right(-120)
t.forward(-100)
t.right(-60)
t.end_fill() # 结束填充
colors = ["red", "orange", "yellow", "green", "blue", "purple"] # 颜色列表
for i in range(len(colors)):
draw_heart(colors[i])
t.left(360 // len(colors)) # 每次循环结束后转动turtle,显示不同的方向
turtle.done() # 运行直到关闭窗口
```
这个代码会在一个彩色的心形序列中循环绘制,每次循环都会改变颜色。如果你想让颜色变化更动态,可以考虑使用时间间隔(`time.sleep()`)或动画效果。
python动态爱心镂空代码跳动
在Python中制作动态爱心镂空图案并使其跳动通常涉及到动画效果和图形处理库,比如`PIL`(Python Imaging Library)或更现代的`pygame`。以下是一个简单的例子,使用`turtle`模块创建一个动态爱心形状:
```python
import turtle
# 创建画笔
t = turtle.Turtle()
t.speed(0) # 设置快速绘制
t.hideturtle() # 隐藏画笔
# 定义爱心函数
def draw_heart(x, y):
t.penup()
t.goto(x, y)
t.pendown()
for _ in range(2):
t.begin_fill()
t.forward(140)
t.right(145)
t.forward(70)
t.right(90)
t.forward(140)
t.right(145)
t.end_fill()
t.penup()
t.left(90)
t.forward(28)
t.pendown()
# 演示爱心跳动
for i in range(10): # 可调整次数以增加跳动频率
t.color("red") # 爱心颜色
draw_heart(-100 + i * 60, -100) # 跳跃位置
turtle.update() # 更新画面
t.penup()
t.setposition(-100, -100) # 每次跳完回到原点
turtle.sleep(0.5) # 延迟一段时间
turtle.done() # 关闭窗口前等待用户按下退出键
```
在这个代码里,爱心会在屏幕中心按照一定的步长向上跳跃,并改变颜色,模拟出一种跳动的效果。
阅读全文
相关推荐
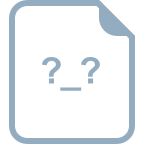
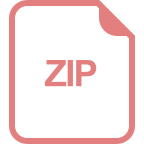












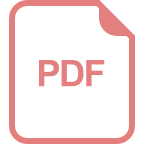