C语言hello,world
时间: 2023-08-17 07:05:00 浏览: 137
### 回答1:
在 C 语言中,可以使用下面的代码输出 "hello, world":
```
#include <stdio.h>
int main(void) {
printf("hello, world\n");
return 0;
}
```
需要注意的是,"#include <stdio.h>" 是在引用标准输入/输出库,"int main(void)" 是程序的入口函数,"printf" 函数用于将字符串输出到控制台,"\n" 是换行符。
### 回答2:
"Hello, world!" 是一个经典的C语言程序,它是初学者通常接触到的第一个C语言程序,也是入门必学的一段代码。
C语言是一种高级编程语言,由贝尔实验室的Dennis M. Ritchie于1972年开发。它是一种通用的编程语言,被广泛应用于计算机科学和软件开发领域。
"Hello, world!"程序是一个非常简单的示例程序,用于展示C语言的基本语法和输出功能。下面是一个使用C语言编写的"Hello, world!"程序的代码:
```c
#include <stdio.h>
int main()
{
printf("Hello, world!\n");
return 0;
}
```
以上代码中,`#include <stdio.h>`是引入标准输入输出库,`int main()`是程序的入口函数。`printf("Hello, world!\n");`是输出函数,用于将字符串"Hello, world!"打印到屏幕上。`return 0;`表示程序成功执行并返回0。
要执行这个程序,需要在C语言编译器中进行编译和执行。首先,将代码保存为一个以`.c`为扩展名的文件,比如`helloworld.c`。然后使用编译器将源代码编译成可执行文件。在命令行中,可以使用以下命令进行编译和执行:
```
$ gcc helloworld.c -o helloworld
$ ./helloworld
```
输出结果将会显示为:
```
Hello, world!
```
"Hello, world!"程序虽然简单,但它是学习C语言的重要一步。通过编写和运行这个简单的程序,可以帮助初学者熟悉C语言的基本语法和程序的编译执行过程。
### 回答3:
C语言的hello, world程序是一种非常简单的程序,它是许多编程语言中的传统入门程序。这个程序的目的是输出屏幕上的“hello, world”这句话。
在C语言中,要打印输出信息到屏幕上,可以使用printf函数。下面是一个简单的C语言程序,实现了输出“hello, world”的功能:
```c
#include <stdio.h> // 包含了用于输入输出的库
int main() // 主函数,程序从这里开始执行
{
printf("hello, world\n"); // 使用printf函数打印输出信息
return 0; // 返回0表示程序执行成功
}
```
在这段代码中,我们首先使用#include指令包含了stdio.h头文件,该头文件里包含了printf函数的声明。然后,我们定义了一个名为main的函数作为程序的入口,它没有参数并且返回一个整数值。
在main函数中,我们使用printf函数打印输出了“hello, world”。printf函数的参数是一个字符串常量,它需要用双引号括起来。
最后,我们使用return语句返回了0,表示程序执行成功。
当我们编译并运行这个程序时,屏幕上就会显示出“hello, world”这句话。这个简单的示例程序不仅可以检验编译环境是否正常,而且也可以让我们迅速地入门C语言的基本语法。
相关推荐
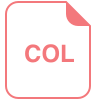
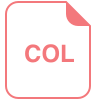
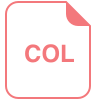
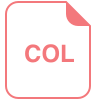
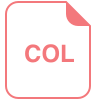









