vue3 ts 自动轮播图片并有切换效果
时间: 2024-02-01 15:04:35 浏览: 143
你可以使用Vue3的Composition API来实现自动轮播图片并有切换效果的功能。下面是一个基本的示例:
```
<template>
<div class="slider">
<div class="slider-container">
<div class="slider-item" v-for="(item, index) in images" :key="index" :style="{ backgroundImage: `url(${item})`, left: `${(index - currentIndex) * 100}%` }"></div>
</div>
</div>
</template>
<script lang="ts">
import { ref, onMounted, onUnmounted } from 'vue';
export default {
setup() {
const images = [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg',
'https://example.com/image3.jpg'
];
const currentIndex = ref(0);
let interval: any = null;
const startAutoSlide = () => {
interval = setInterval(() => {
currentIndex.value = (currentIndex.value + 1) % images.length;
}, 3000);
};
const stopAutoSlide = () => {
clearInterval(interval);
};
onMounted(() => {
startAutoSlide();
});
onUnmounted(() => {
stopAutoSlide();
});
return {
images,
currentIndex,
startAutoSlide,
stopAutoSlide
};
}
};
</script>
<style scoped>
.slider {
width: 100%;
overflow: hidden;
position: relative;
}
.slider-container {
width: 300%;
display: flex;
transition: left 0.5s ease-in-out;
}
.slider-item {
width: 33.3333%;
height: 200px;
background-size: cover;
background-position: center;
position: absolute;
top: 0;
left: 0;
}
</style>
```
在这个示例中,我们使用了一个`currentIndex`的ref来保存当前显示图片的索引。使用`setInterval`函数来定期更新`currentIndex`的值,达到自动轮播的效果。同时,我们将图片放置在一个横向滚动的容器中,使用`left`样式属性来控制当前显示的图片位置。
你可以根据自己的需要修改样式和定时器的时间间隔来实现更自定义的效果。
阅读全文
相关推荐
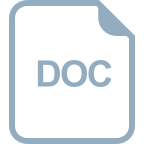
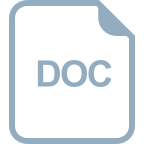
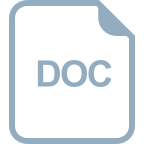

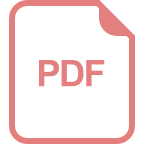
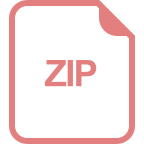
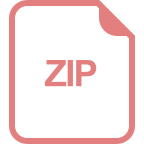
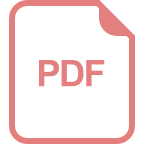
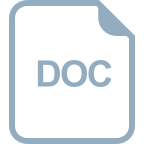
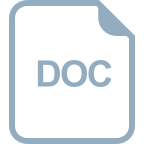
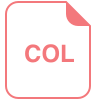
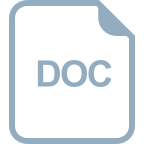
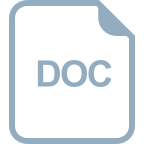
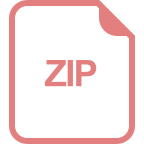
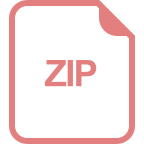
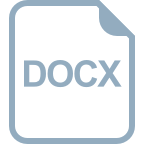