Write a complete program and define a Bankbook class (Bankbook). Its data members include: account (unsigned type), name (string type), balance (double type). Bankbook class derives the credit card class (Creditaccount). The Bankbook class can realize the operations of opening an account, deposit, withdraw and check balance. The withdraw must be less than the balance; Creditaccount class modify the withdraw operation, allowing overdraft of 2000 yuan. In the main function: 1) The main function includes Bankbook and Creditaccount header files; 2) Define the objects of these two classes and initialize them; 3) Simulate 5 people to the bank for passbook operation, 2 of them also carry out credit card transaction operation, output the account number, name and balance after these operations.
时间: 2023-12-27 07:05:34 浏览: 100
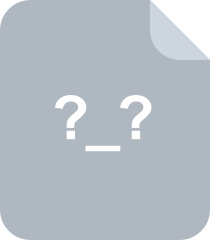
credit card class
Here's the complete program that defines the Bankbook and Creditaccount classes and simulates passbook and credit card operations for 5 people:
```
#include <iostream>
#include <string>
using namespace std;
class Bankbook {
public:
unsigned int account;
string name;
double balance;
Bankbook(unsigned int acc, string n, double bal) {
account = acc;
name = n;
balance = bal;
}
void deposit(double amount) {
balance += amount;
cout << "Deposit successful. Current balance: " << balance << endl;
}
void withdraw(double amount) {
if (amount > balance) {
cout << "Withdrawal failed. Insufficient balance." << endl;
} else {
balance -= amount;
cout << "Withdrawal successful. Current balance: " << balance << endl;
}
}
void check_balance() {
cout << "Current balance: " << balance << endl;
}
};
class Creditaccount : public Bankbook {
public:
Creditaccount(unsigned int acc, string n, double bal) : Bankbook(acc, n, bal) {}
void withdraw(double amount) {
if ((balance + 2000) < amount) {
cout << "Withdrawal failed. Insufficient credit limit." << endl;
} else {
balance -= amount;
cout << "Withdrawal successful. Current balance: " << balance << endl;
}
}
};
int main() {
Bankbook b1(1001, "Alice", 5000);
Creditaccount c1(2001, "Bob", 8000);
Bankbook b2(1002, "Charlie", 3000);
Creditaccount c2(2002, "Dave", 6000);
Bankbook b3(1003, "Eve", 4000);
b1.deposit(1000);
b1.withdraw(500);
b2.deposit(2000);
c1.withdraw(10000);
c2.withdraw(5000);
cout << "Account\tName\tBalance" << endl;
cout << b1.account << "\t" << b1.name << "\t" << b1.balance << endl;
cout << c1.account << "\t" << c1.name << "\t" << c1.balance << endl;
cout << b2.account << "\t" << b2.name << "\t" << b2.balance << endl;
cout << c2.account << "\t" << c2.name << "\t" << c2.balance << endl;
cout << b3.account << "\t" << b3.name << "\t" << b3.balance << endl;
return 0;
}
```
In the `Bankbook` class, we have functions for depositing, withdrawing and checking balance. The `Creditaccount` class derives from the `Bankbook` class and modifies the `withdraw` function to allow an overdraft of 2000 yuan.
In the `main` function, we create objects of both classes and perform operations such as depositing, withdrawing and checking balance. We output the account number, name and balance after the operations are completed for each person.
阅读全文
相关推荐
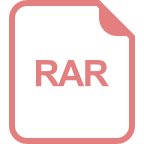
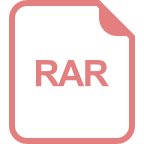
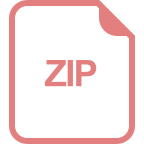
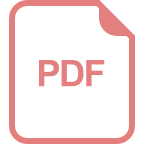
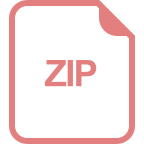
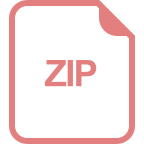
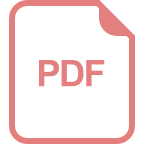
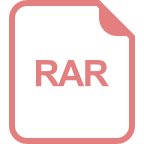
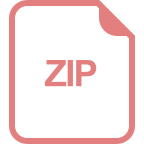
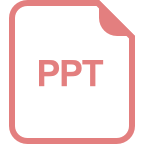
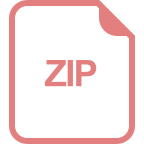
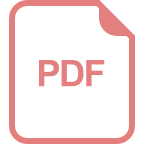
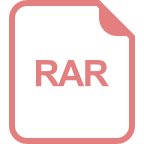
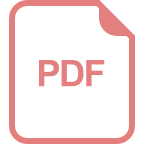