idle python五子棋人机对战源码
时间: 2023-06-18 21:03:20 浏览: 163
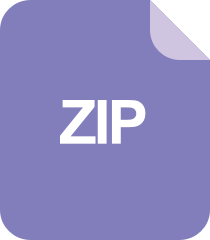
基于python的五子棋.zip
以下是使用Python实现的五子棋人机对战的源代码:
```python
import random
# 初始化棋盘
def new_board():
board = []
for i in range(15):
board.append(['+']*15)
return board
# 打印棋盘
def print_board(board):
print(' 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15')
for i in range(15):
print(str(i+1)+' '+' '.join(board[i]))
# 判断是否胜利
def check_win(board, x, y, player):
count = 1
# 横向
for i in range(1,5):
if y-i < 0 or board[x][y-i] != player:
break
count += 1
for i in range(1,5):
if y+i > 14 or board[x][y+i] != player:
break
count += 1
if count >= 5:
return True
count = 1
# 纵向
for i in range(1,5):
if x-i < 0 or board[x-i][y] != player:
break
count += 1
for i in range(1,5):
if x+i > 14 or board[x+i][y] != player:
break
count += 1
if count >= 5:
return True
count = 1
# 左上到右下
for i in range(1,5):
if x-i < 0 or y-i < 0 or board[x-i][y-i] != player:
break
count += 1
for i in range(1,5):
if x+i > 14 or y+i > 14 or board[x+i][y+i] != player:
break
count += 1
if count >= 5:
return True
count = 1
# 左下到右上
for i in range(1,5):
if x+i > 14 or y-i < 0 or board[x+i][y-i] != player:
break
count += 1
for i in range(1,5):
if x-i < 0 or y+i > 14 or board[x-i][y+i] != player:
break
count += 1
if count >= 5:
return True
return False
# 人下棋
def player_move(board):
while True:
try:
x,y = map(int, input('请输入下棋坐标,用空格分隔(例如:1 1):').split())
if board[x-1][y-1] != '+':
print('该位置已经有棋子了,请重新输入!')
continue
board[x-1][y-1] = 'X'
return
except:
print('输入有误,请重新输入!')
# AI下棋
def ai_move(board):
# 随机下棋
while True:
x = random.randint(0, 14)
y = random.randint(0, 14)
if board[x][y] == '+':
board[x][y] = 'O'
print('AI下棋坐标:', x+1, y+1)
return
# 主函数
def main():
board = new_board()
print_board(board)
while True:
player_move(board)
print_board(board)
if check_win(board, player_move_x-1, player_move_y-1, 'X'):
print('恭喜你,你赢了!')
break
ai_move(board)
print_board(board)
if check_win(board, ai_move_x, ai_move_y, 'O'):
print('很遗憾,你输了!')
break
if __name__ == '__main__':
main()
```
以上代码可以在Python的IDLE环境下运行。在游戏开始时,程序会要求玩家输入下棋的坐标,然后打印出棋盘,并等待AI下棋。游戏结束时,程序会根据胜利者输出相应的信息。
阅读全文
相关推荐
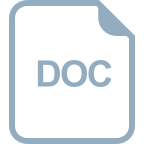
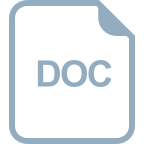
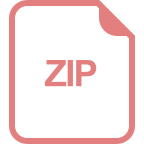
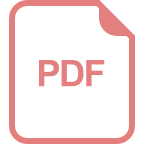
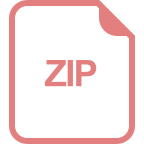
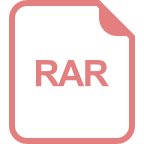
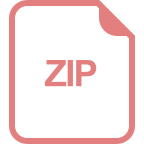
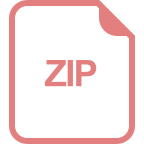
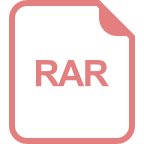
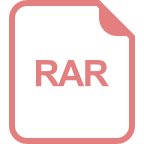
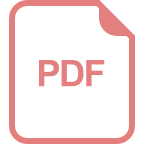
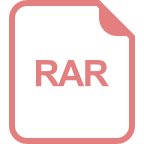
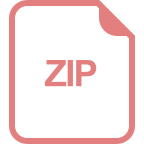
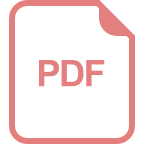
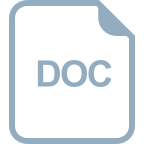