java使用swagger
时间: 2023-09-05 07:11:38 浏览: 134
在Java中使用Swagger可以通过以下步骤来实现:
1. 在你的Maven或Gradle项目中添加Swagger的依赖项。例如,对于Maven项目,可以在`pom.xml`文件中添加以下内容:
```xml
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
```
2. 创建一个配置类来启用Swagger。在该类上使用`@EnableSwagger2`注解,如下所示:
```java
import org.springframework.context.annotation.Configuration;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
@Configuration
@EnableSwagger2
public class SwaggerConfig {
}
```
3. 创建一个API控制器类,并使用Swagger的注解来描述API接口。例如:
```java
import org.springframework.web.bind.annotation.*;
import io.swagger.annotations.*;
@RestController
@RequestMapping("/api")
@Api(tags = "示例API")
public class SampleController {
@GetMapping("/hello")
@ApiOperation("获取欢迎信息")
public String hello() {
return "Hello, Swagger!";
}
@PostMapping("/user")
@ApiOperation("创建用户")
public User createUser(@RequestBody User user) {
// 处理创建用户的逻辑
return user;
}
// 其他API接口...
}
```
4. 启动你的应用程序,并访问Swagger UI界面。Swagger UI界面默认可以通过`http://localhost:8080/swagger-ui.html`进行访问。你将能够看到由Swagger自动生成的API文档,并可以在界面上进行交互测试和调试。
请注意,上述示例中使用了Spring Boot和Springfox Swagger来演示使用Swagger的过程。你可以根据你的项目框架和需要进行相应的调整。另外,还可以通过注解来进一步定制API文档的展示效果和描述信息。
希望这些步骤能够帮助你在Java项目中成功使用Swagger!
阅读全文
相关推荐
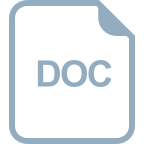
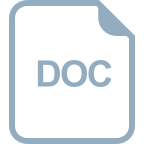
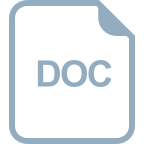
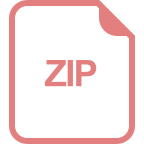

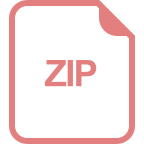
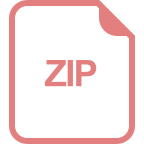
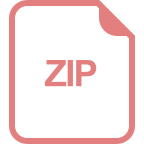
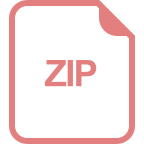
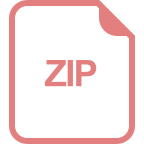
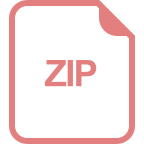
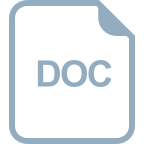
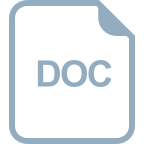
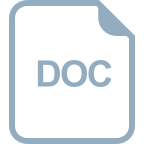
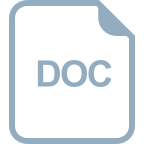
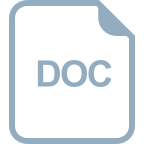


