VUE3+TS+VITE 将input输入的内容格式化将其格式化为 (xxx) xxx-xxxx 的电话号码并限制输入长度
时间: 2024-03-12 20:50:08 浏览: 18
好的,以下是使用 Vue3 + TypeScript + Vite 实现将输入的内容格式化为电话号码的示例代码:
```vue
<template>
<div>
<label for="phone-input">Phone Number:</label>
<input id="phone-input" type="tel" v-model="phone" @input="handleInput" :maxlength="14" />
</div>
</template>
<script lang="ts">
import { defineComponent, ref } from 'vue';
export default defineComponent({
name: 'PhoneInput',
setup() {
const phone = ref('');
const handleInput = (event: InputEvent) => {
const input = (event.target as HTMLInputElement).value.replace(/\D/g, '').substring(0, 10); // 只保留数字,限制长度为10
const formattedInput = input.replace(/^(\d{3})(\d{0,3})(\d{0,4}).*/, '($1) $2-$3'); // 格式化为 (xxx) xxx-xxxx
phone.value = formattedInput;
};
return {
phone,
handleInput,
};
},
});
</script>
```
在上面的代码中,我们使用了 Vue3 的 composition API,通过 `defineComponent` 定义了一个名为 `PhoneInput` 的组件。在组件中,我们使用了 `ref` 来定义了一个响应式数据 `phone`,用于保存用户输入的电话号码。然后,我们定义了一个名为 `handleInput` 的函数,用于处理用户输入的内容。
在 `handleInput` 函数中,我们使用了 `event.target` 来获取当前的输入框元素,并使用 `replace` 函数去掉非数字字符,并使用 `substring` 函数限制长度为 10。然后,我们使用 `replace` 函数将数字格式化为电话号码的形式,并将格式化后的电话号码设置为 `phone` 的值。
最后,我们在模板中使用 `v-model` 来实现双向数据绑定,将 `phone` 的值绑定到输入框的值上,并通过 `@input` 事件监听器来调用 `handleInput` 函数,实时处理用户的输入。同时,我们通过 `:maxlength` 属性来限制输入框的长度为 14,包括括号和破折号。
希望这个回答能够帮助你解决问题!
相关推荐
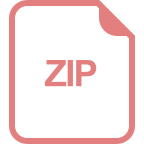
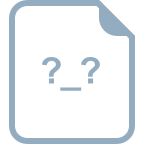














