编程:创建两个线程,要求如下: (1) 一个线程输出1~26,另一个线程输出 A~Z (2)一个线程使用继承Thread类的写法,另一个线程使用实现Runnable接口的写法用java写
时间: 2024-11-22 11:28:46 浏览: 22
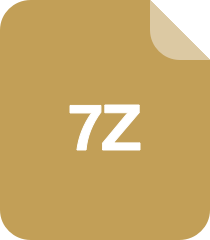
多线程设计-四个按钮控制两个计时器的开始和关闭.7z
在Java中,可以使用两种方式创建并启动线程来满足这个需求:通过继承`Thread`类和实现`Runnable`接口。
**使用继承Thread类的方式**:
```java
class ThreadOutput implements Runnable {
private int number;
private char letter;
public ThreadOutput(int start, char firstChar) {
this.number = start;
this.letter = firstChar;
}
@Override
public void run() {
for (int i = number; i <= 26; i++) {
System.out.println("Number " + i);
}
while (number < 26) {
System.out.print(letter++);
}
}
}
public class Main {
public static void main(String[] args) {
Thread thread1 = new Thread(new ThreadOutput(1, 'A'));
thread1.start();
// 同理创建第二个线程,如thread2,处理B到Z的输出
}
}
```
**使用实现Runnable接口的方式**:
```java
class ThreadOutput implements Runnable {
private int number;
private char letter;
public ThreadOutput(int start, char firstChar) {
this.number = start;
this.letter = firstChar;
}
@Override
public void run() {
for (int i = number; i <= 26; i++) {
System.out.println("Number " + i);
}
while (number < 26) {
System.out.print(letter++);
}
}
}
public class Main {
public static void main(String[] args) {
Thread thread1 = new Thread(new ThreadOutput(1, 'A'));
thread1.start();
Thread thread2 = new Thread(new ThreadOutput(0, 'B')); // 使用不同的初始化值处理第二个线程
thread2.start();
}
}
```
在这个例子中,每个线程都有自己的`run()`方法,分别负责打印数字和字母序列。需要注意的是,为了区分两个线程的输出,这里假设`thread1`从1开始数到26,`thread2`从'A'开始到'Z'。
阅读全文
相关推荐
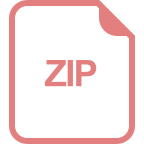
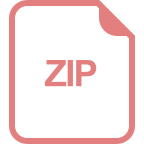






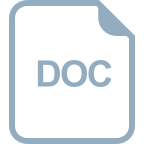
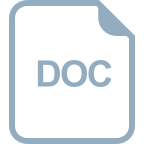
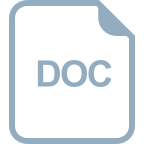
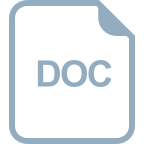
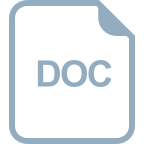
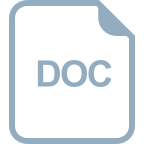
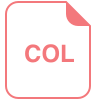
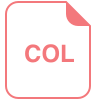


