RS485接收数据并显示在lcd1602上的c语言代码
时间: 2023-09-22 20:07:38 浏览: 87
以下是一个简单的示例代码,可以通过RS485接收数据并在LCD1602上显示:
```c
#include <reg52.h>
#include <stdio.h>
#include <string.h>
sbit RS485EN = P1^0;
sbit RS485RW = P1^1;
sbit LCD_RS = P2^0;
sbit LCD_RW = P2^1;
sbit LCD_EN = P2^2;
unsigned char g_uart_buf[256]={0};
unsigned char g_uart_cnt=0;
void InitUART(void);
void InitLCD(void);
void LCD1602_WriteCmd(unsigned char cmd);
void LCD1602_WriteData(unsigned char dat);
void LCD1602_WriteString(unsigned char x, unsigned char y, unsigned char *s);
void RS485_Send(unsigned char *buf, unsigned char len);
void RS485_Recv(unsigned char *buf);
void main()
{
unsigned char buf[256]={0};
unsigned char len=0;
unsigned char i=0;
InitUART();
InitLCD();
while(1)
{
RS485_Recv(buf);
len=strlen(buf);
LCD1602_WriteString(0, 0, "Recv:");
for(i=0;i<len;i++)
{
LCD1602_WriteData(buf[i]);
}
}
}
void InitUART(void)
{
TMOD |= 0x20; //设置定时器1为模式2
TH1 = 0xfd; //设定波特率为9600bps
TL1 = 0xfd;
SCON = 0x50; //设置串口为模式1,允许接收中断
ES = 1; //允许串口中断
TR1 = 1; //启动定时器1
}
void InitLCD(void)
{
LCD1602_WriteCmd(0x38); //设置16*2显示,5*7点阵,8位数据总线
LCD1602_WriteCmd(0x0c); //开显示,关光标,不闪烁
LCD1602_WriteCmd(0x06); //文字不动,光标自动右移
LCD1602_WriteCmd(0x01); //清屏
}
void LCD1602_WriteCmd(unsigned char cmd)
{
RS485EN = 0;
LCD_RS = 0;
LCD_RW = 0;
LCD_EN = 0;
LCD_EN = 1;
LCD_EN = 0;
P0 = cmd;
delay_ms(1);
}
void LCD1602_WriteData(unsigned char dat)
{
RS485EN = 0;
LCD_RS = 1;
LCD_RW = 0;
LCD_EN = 0;
LCD_EN = 1;
LCD_EN = 0;
P0 = dat;
delay_ms(1);
}
void LCD1602_WriteString(unsigned char x, unsigned char y, unsigned char *s)
{
unsigned char addr=0;
if(x==0) addr=0x80+y; //第一行
else addr=0xc0+y; //第二行
LCD1602_WriteCmd(addr);
while(*s)
{
LCD1602_WriteData(*s++);
}
}
void RS485_Send(unsigned char *buf, unsigned char len)
{
unsigned char i=0;
RS485EN = 1;
RS485RW = 0;
for(i=0;i<len;i++)
{
SBUF = buf[i];
while(!TI);
TI = 0;
}
RS485EN = 0;
}
void RS485_Recv(unsigned char *buf)
{
while(RI==0);
RI = 0;
buf[g_uart_cnt++]=SBUF;
if(g_uart_cnt>=255) g_uart_cnt=0;
}
```
以上代码中,使用了RS485EN和RS485RW两个控制引脚来控制RS485芯片的发送和接收模式。在主函数中,不断调用RS485_Recv函数来接收数据,然后将数据显示在LCD1602上。
需要注意的是,以上代码仅供参考,具体的实现方式可能因为硬件和软件环境的不同而有所差异。
阅读全文
相关推荐
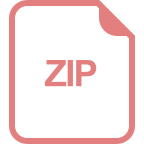
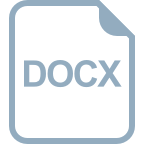
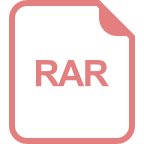
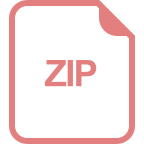
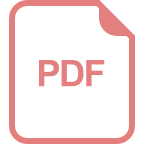
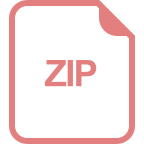
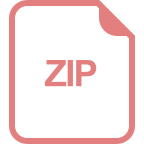
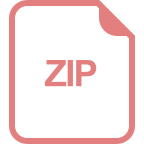
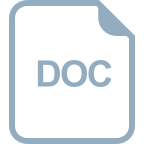
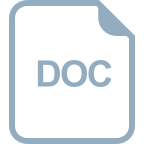
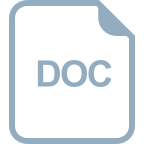
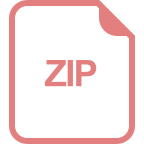