html转json
时间: 2023-07-19 21:20:47 浏览: 55
将 HTML 转换为 JSON 的过程可以分为两个步骤:
1. 解析 HTML:使用解析器(如 Beautiful Soup)将 HTML 转换为 Python 对象(如 BeautifulSoup 对象)。
2. 转换为 JSON:使用 Python 的内置模块 json 将 Python 对象转换为 JSON 格式。
下面是一个示例代码,它演示了如何将 HTML 转换为 JSON:
```python
from bs4 import BeautifulSoup
import json
# 要转换为 JSON 的 HTML
html = '<html><body><p>Hello, world!</p></body></html>'
# 解析 HTML
soup = BeautifulSoup(html, 'html.parser')
# 将 BeautifulSoup 对象转换为 JSON
json_data = json.dumps(soup.prettify())
# 输出 JSON
print(json_data)
```
在此示例中,我们使用 Beautiful Soup 解析 HTML,并使用 json 模块将 BeautifulSoup 对象转换为 JSON。请注意,我们使用 prettify() 方法来获取漂亮的 HTML 字符串,这样我们就可以将其转换为 JSON。
相关问题
html 转 json
将 HTML 转换为 JSON 可能涉及到多种方法和工具,具体取决于 HTML 的结构和目的。下面是一个示例,使用 Python 的 BeautifulSoup 库和 json 库将 HTML 转换为 JSON。
```python
from bs4 import BeautifulSoup
import json
# 假设我们有以下 HTML 字符串
html_str = "<html><body><h1>Hello world!</h1><p>This is a paragraph.</p></body></html>"
# 解析 HTML
soup = BeautifulSoup(html_str, 'html.parser')
# 将 HTML 结构转换为嵌套字典
def html_to_dict(element):
d = {}
d['name'] = element.name
if element.attrs:
d['attrs'] = element.attrs
if element.string:
d['string'] = element.string
d['children'] = [html_to_dict(e) for e in element.contents if e.name is not None]
return d
html_dict = html_to_dict(soup)
# 将字典转换为 JSON
html_json = json.dumps(html_dict)
print(html_json)
```
输出的 JSON 如下所示:
```json
{
"name": "html",
"attrs": {},
"children": [
{
"name": "body",
"attrs": {},
"children": [
{
"name": "h1",
"attrs": {},
"children": [
{
"string": "Hello world!",
"children": []
}
]
},
{
"name": "p",
"attrs": {},
"children": [
{
"string": "This is a paragraph.",
"children": []
}
]
}
]
}
]
}
```
cheerio html转json
可以使用 `cheerio` 和 `htmlparser2` 库将 HTML 转换为 JSON。
首先需要安装这两个库:
```
npm install cheerio htmlparser2
```
接着可以使用以下代码将 HTML 转换为 JSON:
```javascript
const cheerio = require('cheerio');
const htmlparser2 = require('htmlparser2');
const html = '<div><h1>Title</h1><p>Content</p></div>';
const $ = cheerio.load(html);
const json = {};
const parseChildren = (element, parentJson) => {
const children = $(element).children();
if (children.length === 0) {
parentJson[element.name] = $(element).text();
} else {
parentJson[element.name] = {};
children.each((i, el) => {
parseChildren(el, parentJson[element.name]);
});
}
};
parseChildren($.root()[0], json);
console.log(json);
```
这个代码将输出以下 JSON:
```json
{
"div": {
"h1": "Title",
"p": "Content"
}
}
```
这个 JSON 表示了原始 HTML 中的层级关系和文本内容。
相关推荐
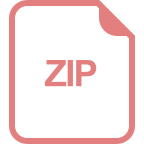
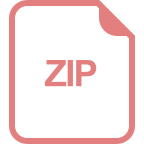
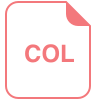
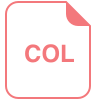
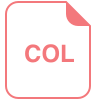









