用c语言写出strcpy和strcat函数,不用指针
时间: 2023-11-28 14:15:44 浏览: 54
好的,这是两个字符串操作函数的代码实现:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void myStrcpy(char dest[], char src[]) {
int i = 0;
while (src[i] != '\0') {
dest[i] = src[i];
i++;
}
dest[i] = '\0';
}
void myStrcat(char dest[], char src[]) {
int i = 0, j = 0;
while (dest[i] != '\0') {
i++;
}
while (src[j] != '\0') {
dest[i] = src[j];
i++;
j++;
}
dest[i] = '\0';
}
int main() {
char str1[100] = "Hello";
char str2[100] = "World";
char str3[100] = "Goodbye";
char str4[100] = "Cruel World";
myStrcpy(str1, str2);
printf("%s\n", str1);
myStrcat(str3, str4);
printf("%s\n", str3);
return 0;
}
```
在上面的代码中,`myStrcpy()` 函数实现了字符串的复制,它接受两个参数:目标字符串和源字符串。函数使用一个循环通过逐个字符复制源字符串中的字符到目标字符串中,直到遇到空字符为止。最后,在目标字符串的末尾添加一个空字符以终止字符串。
`myStrcat()` 函数实现了字符串的连接,它也接受两个参数:目标字符串和源字符串。函数使用两个循环,第一个循环找到目标字符串的末尾,第二个循环将源字符串中的字符逐个添加到目标字符串中。最后,在目标字符串的末尾添加一个空字符以终止字符串。
这些函数的实现方式可能不太高效,但它们没有使用指针,符合了您的要求。
相关推荐
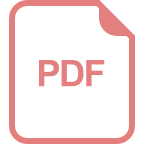
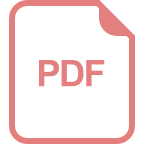
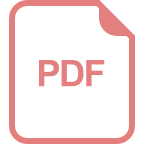














