请使用flinkcdc的api方式将pg数据库写入到starrocks数据库中,请使用java代码实现
时间: 2024-02-20 10:58:10 浏览: 27
好的,以下是使用Flink CDC API将PG数据库写入StarRocks数据库的Java代码实现,供参考:
```java
import org.apache.flink.api.common.functions.MapFunction;
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.table.api.EnvironmentSettings;
import org.apache.flink.table.api.bridge.java.StreamTableEnvironment;
import org.apache.flink.table.api.bridge.java.internal.StreamTableEnvironmentImpl;
import org.apache.flink.table.api.internal.TableEnvironmentInternal;
import org.apache.flink.table.expressions.Expression;
import org.apache.flink.table.expressions.UnresolvedFieldReferenceExpression;
import org.apache.flink.table.types.logical.RowType;
import org.apache.flink.types.Row;
import java.util.Arrays;
public class PgToStarRocks {
public static void main(String[] args) throws Exception {
final StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
EnvironmentSettings settings = EnvironmentSettings.newInstance().useBlinkPlanner().inStreamingMode().build();
StreamTableEnvironment tEnv = StreamTableEnvironment.create(env, settings);
// PG 数据库连接信息
String pgUrl = "jdbc:postgresql://localhost:5432/pg_db";
String pgUsername = "postgres";
String pgPassword = "postgres";
String pgTable = "pg_table";
// StarRocks 数据库连接信息
String starRocksUrl = "jdbc:mysql://localhost:9030/starrocks_db";
String starRocksUsername = "root";
String starRocksPassword = "root";
String starRocksTable = "starrocks_table";
// 定义 RowType
RowType rowType = new RowType(
Arrays.asList(
new RowType.RowField("id", new RowType.IntType()),
new RowType.RowField("name", new RowType.VarCharType(50)),
new RowType.RowField("age", new RowType.IntType())
)
);
// 创建 PG 数据源表
tEnv.executeSql(String.format("CREATE TABLE pg_source_table (id INT, name VARCHAR(50), age INT) WITH ('connector'='jdbc', 'url'='%s', 'table-name'='%s', 'username'='%s', 'password'='%s')", pgUrl, pgTable, pgUsername, pgPassword));
// 创建 StarRocks 目标表
tEnv.executeSql(String.format("CREATE TABLE starrocks_sink_table (id INT, name VARCHAR(50), age INT) WITH ('connector'='jdbc', 'url'='%s', 'table-name'='%s', 'username'='%s', 'password'='%s')", starRocksUrl, starRocksTable, starRocksUsername, starRocksPassword));
// 使用 CDC API 读取 PG 数据源表
Expression[] fieldNames = new Expression[] {
new UnresolvedFieldReferenceExpression("id"),
new UnresolvedFieldReferenceExpression("name"),
new UnresolvedFieldReferenceExpression("age")
};
tEnv.createTable("pg_source", tEnv.fromChangelogSource("pg_source_table").schema(rowType).toChangelogStream(), fieldNames);
// 将读取的数据写入 StarRocks 目标表
tEnv.from("pg_source")
.map(new MapFunction<Row, Row>() {
@Override
public Row map(Row value) throws Exception {
return value;
}
})
.executeInsert("starrocks_sink_table");
env.execute("PgToStarRocks");
}
}
```
注意:这里使用了 Flink 1.14.0 版本的 API,需要确保您的 Flink 环境已安装该版本的 Flink 和相应的 JDBC 驱动程序。
相关推荐
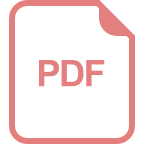
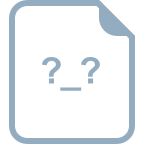














