python定时发送邮件outlook
时间: 2024-12-11 19:13:43 浏览: 8
在Python中,我们可以使用`smtplib`和`email`库来实现定时发送邮件的功能,这里以Outlook作为电子邮件客户端为例,需要安装`python-outlook`库。以下是基本步骤:
1. 安装所需库:
```bash
pip install python-dateutil
pip install python-outlook
```
2. 导入必要的模块并设置环境变量(如果你的Outlook配置了安全套接层SSL/TLS):
```python
import schedule
import time
from datetime import datetime, timedelta
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from outlook.office365.com import Account
```
3. 创建一个函数来编写和发送邮件:
```python
def send_email():
# 邮件内容、发件人、收件人、主题等信息
sender_email = "your_email@example.com"
password = "your_password" # Outlook授权码或SMTP密码
recipient_email = "recipient_email@example.com"
msg = MIMEMultipart()
msg['From'] = sender_email
msg['To'] = recipient_email
msg['Subject'] = "定时邮件"
body = f"这是一封由Python定时发送的邮件,当前时间是{datetime.now()}"
msg.attach(MIMEText(body, 'plain'))
# 使用Office365身份验证连接Outlook
account = Account(
auth_type='basic',
username=sender_email,
password=password
)
# 连接到SMTP服务器
server = smtplib.SMTP('smtp.office365.com', 587)
server.starttls()
server.login(sender_email, password)
# 发送邮件
server.send_message(msg)
server.quit()
print("邮件已成功发送")
# 定义发送邮件的时间间隔,例如每小时一次
schedule.every(1).hours.do(send_email)
# 开始运行任务调度
while True:
schedule.run_pending()
time.sleep(1)
# 如果你想让程序在达到某个特定时间点后停止,可以添加条件判断如:
# if datetime.now().time() > datetime.strptime('10:30', '%H:%M').time(): break
```
阅读全文
相关推荐
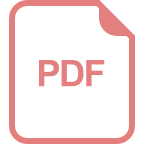
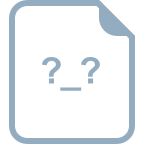
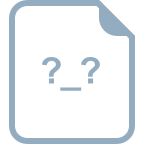















