使用Jupyter实现人脸识别的图像分类代码
时间: 2024-01-06 07:07:15 浏览: 29
好的,以下是使用Jupyter实现人脸识别的图像分类代码:
首先,我们需要导入必要的库和模块:
```python
import numpy as np
import matplotlib.pyplot as plt
import pandas as pd
import seaborn as sns
import cv2
import os
import random
from sklearn.model_selection import train_test_split
from sklearn.metrics import classification_report, confusion_matrix
from keras.models import Sequential
from keras.layers import Conv2D, MaxPooling2D, Dense, Flatten, Dropout
from keras.utils import to_categorical
```
接下来,我们需要加载人脸数据集:
```python
DATADIR = 'path/to/dataset'
CATEGORIES = ['person_1', 'person_2', 'person_3'] # 人脸分类的类别
data = []
for category in CATEGORIES:
path = os.path.join(DATADIR, category)
label = CATEGORIES.index(category)
for img in os.listdir(path):
img_path = os.path.join(path, img)
face_img = cv2.imread(img_path)
face_img = cv2.cvtColor(face_img, cv2.COLOR_BGR2GRAY) # 转换为灰度图像
face_img = cv2.resize(face_img, (50, 50)) # 将图像大小调整为50x50像素
data.append([face_img, label])
random.shuffle(data) # 打乱数据集
```
现在,我们需要将数据集分成训练集和测试集:
```python
X = []
y = []
for face_img, label in data:
X.append(face_img)
y.append(label)
X = np.array(X).reshape(-1, 50, 50, 1) # 调整数据形状
y = to_categorical(y)
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
接下来,我们需要构建卷积神经网络模型:
```python
model = Sequential()
model.add(Conv2D(32, (3, 3), activation='relu', input_shape=X_train.shape[1:]))
model.add(MaxPooling2D((2, 2)))
model.add(Conv2D(64, (3, 3), activation='relu'))
model.add(MaxPooling2D((2, 2)))
model.add(Conv2D(128, (3, 3), activation='relu'))
model.add(MaxPooling2D((2, 2)))
model.add(Flatten())
model.add(Dense(64, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(3, activation='softmax'))
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
```
最后,我们需要训练模型并评估其性能:
```python
history = model.fit(X_train, y_train, epochs=20, validation_data=(X_test, y_test))
y_pred = model.predict(X_test)
y_pred = np.argmax(y_pred, axis=1)
y_test = np.argmax(y_test, axis=1)
print(classification_report(y_test, y_pred))
print(confusion_matrix(y_test, y_pred))
```
这就是使用Jupyter实现人脸识别的图像分类代码了。
相关推荐
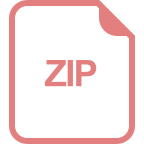
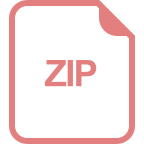
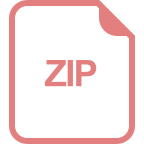














