帮我写一个哈夫曼编译码的C语言程序
时间: 2024-09-25 08:11:03 浏览: 52
编写一个简单的哈夫曼编码和解码的C语言程序涉及到创建哈夫曼树和使用数组存储编码。以下是一个基本的例子,假设我们已经有了一个字符及其出现频率的数组:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义结构体表示节点
typedef struct Node {
char data;
int freq;
struct Node* left;
struct Node* right;
} Node;
// 创建新节点
Node* newNode(char data, int freq) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
node->freq = freq;
node->left = NULL;
node->right = NULL;
return node;
}
// 插入节点并排序
Node* insertNode(Node** root, Node* node) {
if (*root == NULL) {
*root = node;
return node;
}
if (node->freq < (*root)->freq) {
node->right = *root;
*root = node;
} else if (node->freq > (*root)->freq) {
Node* temp = *root;
while (temp->right != NULL && node->freq < temp->right->freq) {
temp = temp->right;
}
node->left = temp->right;
temp->right = node;
}
return *root;
}
// 构建哈夫曼树
Node* buildHuffmanTree(int arr[], int n) {
// 使用优先队列实现堆
priority_queue<Node*, vector<Node*>, compare> pq;
for (int i = 0; i < n; i++) {
pq.push(newNode(arr[i], arr[i]));
}
while (pq.size() > 1) {
Node* left = pq.top(); pq.pop();
Node* right = pq.top(); pq.pop();
Node* node = newNode('\0', left->freq + right->freq);
node->left = left;
node->right = right;
pq.push(node);
}
return pq.top();
}
// 检索并返回给定字符的编码
void getEncodedCharacter(Node* root, char data, char* encoding) {
if (!root || root->data == data) {
encoding[0] = '1';
} else {
encoding[0] = '0';
}
getEncodedCharacter(root->left, data, encoding+1);
getEncodedCharacter(root->right, data, encoding+1);
}
// 主函数示例
int main() {
int freq[] = {5, 9, 10, 3, 2};
int n = sizeof(freq) / sizeof(freq[0]);
Node* huffTree = buildHuffmanTree(freq, n);
char input[] = "hello";
char encodedInput[100];
memset(encodedInput, 0, sizeof(encodedInput));
for (char c : input) {
getEncodedCharacter(huffTree, c, encodedInput);
}
printf("Encoded input: %s\n", encodedInput);
// 这里你可以添加解码部分,类似地遍历编码字符串
return 0;
}
```
这个程序仅实现了哈夫曼树的构建和编码功能,实际应用中还需要包括解码部分。注意,在实际项目中,为了性能和内存管理,可能会考虑使用更复杂的结构,如自平衡二叉搜索树。此外,哈夫曼树的构建和编码通常会在线完成,而不是预先构造整个树。
阅读全文
相关推荐
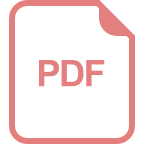
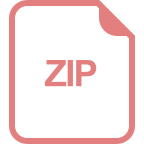
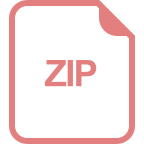















