基于opencv和knn的简单数字识别代码
时间: 2023-06-08 18:05:18 浏览: 78
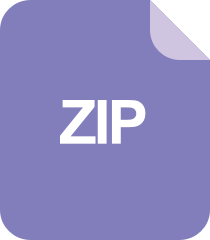
基于opencv的数字识别
请问您需要一个基于opencv和knn的简单数字识别代码的示例吗?如果是的话,以下是一个示例代码:
```
import cv2
import numpy as np
# Load the data for training the KNN classifier
digits = cv2.imread('digits.png', 0)
samples = np.loadtxt('samples.data', np.float32)
responses = np.loadtxt('responses.data', np.float32)
# Create the KNN classifier and train it with the loaded data
model = cv2.ml.KNearest_create()
model.train(samples, cv2.ml.ROW_SAMPLE, responses)
# Load the test image and preprocess it (convert to grayscale, threshold, etc.)
img = cv2.imread('test.png')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
gray = cv2.GaussianBlur(gray, (5, 5), 0)
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY_INV)
thresh = cv2.dilate(thresh, None, iterations=2)
thresh = cv2.erode(thresh, None, iterations=2)
# Find contours in the preprocessed image
contours, hierarchy = cv2.findContours(thresh.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# For each contour, predict the digit using the KNN classifier and draw a rectangle around it
for contour in contours:
(x, y, w, h) = cv2.boundingRect(contour)
if w>=5 and h>=25:
roi = thresh[y:y+h, x:x+w]
roi = cv2.resize(roi, (20, 20))
roi = np.reshape(roi, (1, 400))
roi = np.float32(roi)
ret, results, neighbours, dist = model.findNearest(roi, k=5)
digit = int(results[0][0])
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
cv2.putText(img, str(digit), (x, y-10), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2, cv2.LINE_AA)
# Display the final image
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
该代码使用KNN分类器对预处理后的数字进行分类。该分类器使用已加载和训练的样本数据。在预测数字时,该代码会在数字周围绘制一个矩形以指示数字的位置,并在数字上方绘制数字本身。
阅读全文
相关推荐
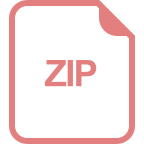
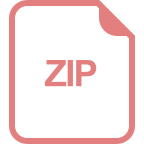

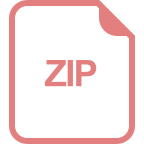
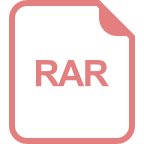
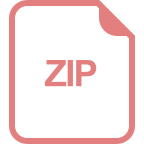
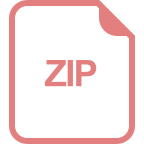
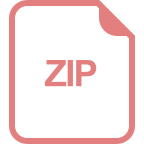
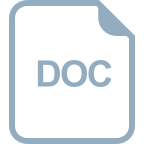



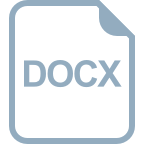