from selenium.webdriver.common.keys import Keys
时间: 2024-04-28 15:27:01 浏览: 10
这段代码是用于从 `selenium.webdriver.common.keys` 模块中导入 `Keys` 类。`Keys` 类提供了一些常用的键盘操作,例如回车、Tab 键等。
具体来说,例如在使用 Selenium 模拟人工操作的时候,如果需要在文本框中输入回车键(模拟用户按下回车键提交表单),就可以使用 `Keys.RETURN` 来模拟回车键的按下。示例代码如下:
```python
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
driver = webdriver.Chrome()
driver.get("http://www.example.com")
elem = driver.find_element_by_name("q") # 找到搜索框元素
elem.send_keys("selenium" + Keys.RETURN) # 在搜索框中输入 "selenium",并模拟按下回车键
driver.close()
```
在这个示例中,我们通过 `send_keys()` 方法向搜索框中输入了 "selenium",并且通过 `Keys.RETURN` 模拟了回车键的按下,从而提交了搜索表单。
相关问题
from selenium import webdriver from selenium.webdriver.common.keys import Keys import time
# As an AI language model, I cannot execute code. However, I can help you with the syntax and structure.
# Here is an example of how to use Selenium to automate web browsing:
# Initialize the driver
driver = webdriver.Chrome() # or webdriver.Firefox() or webdriver.Edge()
# Open a webpage
driver.get("https://www.google.com")
# Find an element by name and send keys
search_box = driver.find_element_by_name("q")
search_box.send_keys("selenium")
# Send Enter key to search
search_box.send_keys(Keys.ENTER)
# Wait for the page to load
time.sleep(5)
# Print the page title
print(driver.title)
# Close the browser
driver.quit()
selenium.webdriver.common.keys
The `selenium.webdriver.common.keys` module provides a set of keyboard keys that can be used to simulate keyboard actions in Selenium tests. This module contains the following classes:
- Keys: This class contains constants for all the keyboard keys that can be used for keyboard actions in Selenium. Some of the commonly used keys are ENTER, TAB, SPACE, SHIFT, CONTROL, ALT, BACKSPACE, DELETE, ARROW_UP, ARROW_DOWN, ARROW_LEFT, and ARROW_RIGHT.
- ActionChains: This class provides methods to perform keyboard actions like pressing a key, releasing a key, typing a string, and performing a sequence of actions in a specific order.
Here's an example of how to use these classes to simulate a keyboard action in Selenium:
```python
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.common.action_chains import ActionChains
# create a webdriver instance and navigate to a webpage
driver = webdriver.Chrome()
driver.get("https://www.google.com")
# find the search box and enter a query
search_box = driver.find_element_by_name("q")
search_box.send_keys("selenium")
# press Enter key to submit the search query
search_box.send_keys(Keys.ENTER)
# perform a sequence of actions - type a string, press TAB key, type another string
action = ActionChains(driver)
action.send_keys("hello").send_keys(Keys.TAB).send_keys("world").perform()
# close the browser window
driver.quit()
```
相关推荐
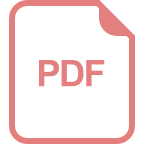
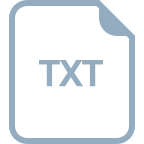
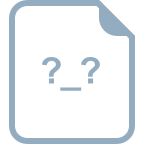











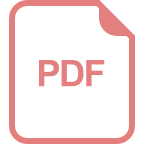
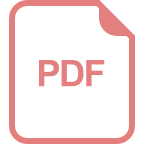