Java中怎么实现mysql中多表关联的查询
时间: 2024-02-13 16:07:37 浏览: 23
在 Java 中实现 mysql 中多表关联查询需要使用 SQL 语句。可以使用 JDBC 连接到 MySQL 数据库,并执行 SQL 查询语句。
下面是一个简单的示例代码,展示了如何在 Java 中执行多表关联查询:
```java
import java.sql.*;
public class MySQLExample {
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost/your_database_name";
static final String USER = "your_username";
static final String PASS = "your_password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try {
Class.forName(JDBC_DRIVER);
System.out.println("Connecting to database...");
conn = DriverManager.getConnection(DB_URL,USER,PASS);
System.out.println("Creating statement...");
stmt = conn.createStatement();
String sql = "SELECT employees.name, departments.dept_name FROM employees " +
"INNER JOIN departments ON employees.dept_id = departments.dept_id";
ResultSet rs = stmt.executeQuery(sql);
while(rs.next()){
String name = rs.getString("name");
String deptName = rs.getString("dept_name");
System.out.print("Name: " + name);
System.out.println(", Department: " + deptName);
}
rs.close();
stmt.close();
conn.close();
} catch(SQLException se) {
se.printStackTrace();
} catch(Exception e) {
e.printStackTrace();
} finally {
try {
if(stmt!=null)
stmt.close();
} catch(SQLException se2) {
}
try {
if(conn!=null)
conn.close();
} catch(SQLException se) {
se.printStackTrace();
}
}
System.out.println("Goodbye!");
}
}
```
在上面的代码中,我们创建了一个连接到 MySQL 数据库的 Connection 对象。然后,我们创建一个 Statement 对象,并使用 SQL 语句执行多表关联查询。
查询结果将被存储在 ResultSet 对象中,我们使用该对象获取查询结果并进行处理。
最后,我们关闭 ResultSet、Statement 和 Connection 对象,释放资源。
注意,上面的代码只是一个简单的示例。在实际开发中,我们应该考虑异常处理、连接池、性能优化等问题。
相关推荐
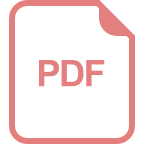
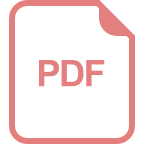
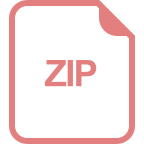














