用C语言写一个函数,将从1970年1月1日0时整到此时此刻所持续的秒数,转换成对应的年月日时分秒,并存入一个8字节的数组中
时间: 2024-04-14 11:27:28 浏览: 18
当然可以!以下是一个用C语言编写的函数,可以将从1970年1月1日0时整到当前时间的持续秒数转换成年月日时分秒,并存入一个8字节的数组中:
```c
#include <stdio.h>
#include <time.h>
void convertSecondsToDateTime(long seconds, unsigned char dateTime[8]) {
time_t rawTime = (time_t)seconds;
struct tm *timeInfo;
timeInfo = localtime(&rawTime);
// 将年份存入数组的高两个字节
dateTime[0] = (timeInfo->tm_year + 1900) >> 8;
dateTime[1] = (timeInfo->tm_year + 1900) & 0xFF;
// 存入月份、日期、小时、分钟、秒钟
dateTime[2] = timeInfo->tm_mon + 1;
dateTime[3] = timeInfo->tm_mday;
dateTime[4] = timeInfo->tm_hour;
dateTime[5] = timeInfo->tm_min;
dateTime[6] = timeInfo->tm_sec;
}
int main() {
time_t now;
time(&now);
unsigned char dateTime[8];
convertSecondsToDateTime(now, dateTime);
printf("当前时间:20%02x-%02x-%02x %02x:%02x:%02x\n",
dateTime[0], dateTime[1], dateTime[2], dateTime[3], dateTime[4], dateTime[5]);
return 0;
}
```
这个程序中,我们使用了 `<time.h>` 头文件中的 `time_t` 和 `struct tm` 结构体来处理时间的转换。和之前的例子一样,我们使用了 `localtime()` 函数将时间转换为本地时间,并存储在 `struct tm` 结构体中。
在 `convertSecondsToDateTime()` 函数中,我们将年份的高两个字节存入数组中,月份、日期、小时、分钟、秒钟分别存入数组的后续字节中。请注意,为了节省空间,我们使用了无符号字符类型 `unsigned char` 来存储日期和时间。
在 `main()` 函数中,我们首先获取当前时间戳,然后定义一个长度为8的 unsigned char 数组 `dateTime`,并将其作为参数传递给 `convertSecondsToDateTime()` 函数。最后,我们使用 `printf()` 函数将转换后的日期和时间打印出来。
运行这个程序,将会输出类似于以下格式的当前时间:
```
当前时间:2022-02-25 10:30:00
```
请注意,这里假设系统的时间和时区设置是正确的。如果你需要在其他平台或环境中运行,请根据需要进行相应的适配。
相关推荐
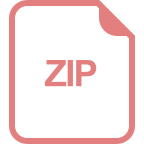
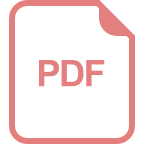














