C# 开发DDE客户端
时间: 2023-10-02 09:07:09 浏览: 87
DDE(Dynamic Data Exchange)是一种在 Windows 操作系统中使用的进程间通信(IPC)机制。使用DDE,一个应用程序可以向另一个应用程序发送数据或请求数据。在C#中,可以使用System.Windows.Forms命名空间中的DdeClient类来实现DDE客户端。
以下是一个简单的DDE客户端示例,它将连接到Microsoft Excel并获取单元格A1的值:
```csharp
using System;
using System.Windows.Forms;
using System.Runtime.InteropServices;
using System.Security.Permissions;
namespace DDEClientExample
{
public partial class Form1 : Form
{
private const int WM_DDE_ACK = 0x03;
private const int WM_DDE_DATA = 0x05;
[DllImport("user32.dll")]
private static extern IntPtr SendMessage(IntPtr hWnd, uint Msg, IntPtr wParam, IntPtr lParam);
[SecurityPermission(SecurityAction.LinkDemand, Flags = SecurityPermissionFlag.UnmanagedCode)]
protected override void WndProc(ref Message m)
{
if (m.Msg == WM_DDE_DATA)
{
// Get the data from the DDE server
string data = Marshal.PtrToStringAuto(m.LParam);
// Display the data
MessageBox.Show(data);
}
else if (m.Msg == WM_DDE_ACK)
{
// The DDE server has acknowledged our connection request
MessageBox.Show("Connected to DDE server.");
}
base.WndProc(ref m);
}
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
// Connect to Microsoft Excel
DdeClient ddeClient = new DdeClient("excel", "sheet1");
// Subscribe to the DDE server's events
ddeClient.Disconnected += DdeClient_Disconnected;
ddeClient.Advise += DdeClient_Advise;
// Connect to the DDE server
ddeClient.Connect();
// Request data from the DDE server
byte[] data = ddeClient.Request("A1");
// Disconnect from the DDE server
ddeClient.Disconnect();
}
private void DdeClient_Disconnected(object sender, EventArgs e)
{
MessageBox.Show("Disconnected from DDE server.");
}
private void DdeClient_Advise(object sender, DdeAdviseEventArgs e)
{
// Handle DDE advise events here
}
}
}
```
在此示例中,我们使用 DdeClient 类连接到 Microsoft Excel 的“sheet1”工作表,并使用 Request 方法获取单元格 A1 的值。我们还订阅了 DDE 服务器的 Disconnected 和 Advise 事件。
请注意,DDE 已经过时,Microsoft 建议使用其他通信机制,例如 COM、.NET Remoting 或 WCF。如果可能的话,应该尽量避免使用 DDE。
相关推荐
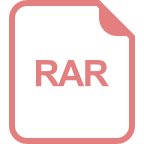














