trie树词频统计,根据频率排序并输出,c语言具体代码
时间: 2023-12-06 14:39:31 浏览: 91
以下是使用 trie 树实现词频统计和排序的 C 语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define ALPHABET_SIZE 26
struct TrieNode {
int frequency; // 该节点对应的词频
struct TrieNode* children[ALPHABET_SIZE]; // 子节点数组
};
// 创建一个新的 Trie 节点
struct TrieNode* createNode() {
struct TrieNode* newNode = (struct TrieNode*) malloc(sizeof(struct TrieNode));
newNode->frequency = 0;
for (int i = 0; i < ALPHABET_SIZE; i++) {
newNode->children[i] = NULL;
}
return newNode;
}
// 插入一个单词到 Trie 树中
void insert(struct TrieNode* root, const char* word) {
int level, index;
int length = strlen(word);
struct TrieNode* pCrawl = root;
for (level = 0; level < length; level++) {
index = word[level] - 'a';
if (!pCrawl->children[index]) {
pCrawl->children[index] = createNode();
}
pCrawl = pCrawl->children[index];
}
pCrawl->frequency++;
}
// 按照词频排序并输出
void sortByFrequency(struct TrieNode* root, char* word, int pos) {
static int wordIndex = 0;
if (root == NULL) {
return;
}
if (root->frequency > 0) {
word[pos] = '\0';
printf("%s - %d\n", word, root->frequency);
}
for (int i = 0; i < ALPHABET_SIZE; i++) {
if (root->children[i] != NULL) {
word[pos] = i + 'a';
sortByFrequency(root->children[i], word, pos+1);
}
}
}
int main() {
struct TrieNode* root = createNode();
char word[100];
// 从标准输入读取单词并插入到 Trie 树中
while (scanf("%s", word) != EOF) {
insert(root, word);
}
// 输出排序后的结果
printf("按照词频排序并输出:\n");
sortByFrequency(root, word, 0);
return 0;
}
```
这个程序可以从标准输入中读取单词并对其进行词频统计和排序,并将结果输出到标准输出中。
阅读全文
相关推荐




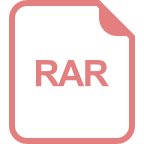






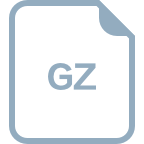

