python调用powershell
时间: 2023-09-07 13:04:32 浏览: 77
### 回答1:
你可以使用 Python 的 subprocess 模块来调用 PowerShell。例如:
```
import subprocess
powerShellCommand = "Write-Host 'Hello from PowerShell'"
# Run the PowerShell command using subprocess.run
result = subprocess.run(["powershell", "-Command", powerShellCommand], capture_output=True, text=True)
# print the output
print(result.stdout)
```
这将在命令行输出 "Hello from PowerShell"。
注意,需要将命令封装在一个字符串中,并使用 `-Command` 参数传递给 PowerShell。
### 回答2:
Python可以通过调用PowerShell来执行一些高级任务,如执行脚本、管理操作系统、设置环境变量等。通过调用PowerShell,Python可以获得更大的灵活性和功能扩展。
要调用PowerShell,可以使用`subprocess`模块。这个模块允许Python与操作系统进行交互,并执行外部命令。我们可以创建一个Python脚本,并在其中使用`subprocess`模块来调用PowerShell。
首先,我们需要导入`subprocess`模块,然后可以使用`subprocess.run()`函数来执行PowerShell命令。下面是一个简单的示例:
```python
import subprocess
# 执行PowerShell命令
result = subprocess.run(["powershell", "Get-Process"], capture_output=True, text=True)
# 输出PowerShell命令的结果
print(result.stdout)
```
在上述示例中,`subprocess.run()`函数接受一个列表作为参数。列表的第一个元素是要执行的命令,后面的元素是命令的参数。
`capture_output=True`参数用于捕获命令的输出结果,`text=True`参数用于指定输出结果为文本格式。
通过上述示例,我们可以获取并打印PowerShell命令"Get-Process"的输出结果。
除了执行简单的命令,我们还可以执行PowerShell脚本、传递变量等。通过这种方式,Python可以与PowerShell进行交互,并实现更加复杂的操作。
总结起来,Python可以通过调用PowerShell来执行一系列操作。使用`subprocess`模块,我们可以执行PowerShell命令,获取结果并进行进一步处理,从而实现更多的功能和灵活性。
### 回答3:
在Python中调用PowerShell可以通过subprocess模块实现。该模块允许我们在Python中启动一个新的进程并与其进行交互。
首先,我们需要导入subprocess模块,然后使用`subprocess.Popen()`函数创建一个新的进程。该函数接受一个命令作为参数,我们可以将要执行的PowerShell命令作为参数传递给该函数。
示例代码如下:
```python
import subprocess
def run_powershell_command(command):
process = subprocess.Popen(["powershell", command], stdout=subprocess.PIPE)
result = process.communicate()[0].decode("utf-8")
return result
powershell_command = 'Write-Output "Hello, World!"'
output = run_powershell_command(powershell_command)
print(output)
```
在上述代码中,我们定义了一个函数`run_powershell_command(command)`,该函数接受一个命令作为参数,并返回命令执行的结果。
在主程序中,我们定义了一个PowerShell命令`'Write-Output "Hello, World!"'`,然后调用`run_powershell_command()`函数来执行该命令,并将其结果输出。
注意,我们使用`subprocess.PIPE`来捕获PowerShell命令的输出,并使用`communicate()`函数获取输出结果。
通过这种方式,我们可以在Python中方便地调用PowerShell命令,并获取其结果。当然,你可以使用更复杂的PowerShell命令和参数,实现更强大的功能。
相关推荐
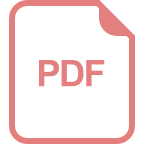
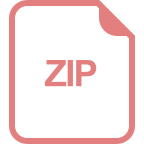
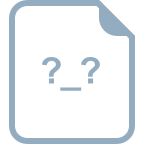
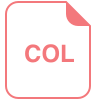













