首页C++ 不存在用户定义的从 type 到std::function<double (std::default_random_engine &)>” 的适当转换
C++ 不存在用户定义的从 type 到std::function<double (std::default_random_engine &)>” 的适当转换
时间: 2024-09-30 18:07:02 浏览: 32
在 C++ 中,`std::function` 是一种通用函数指针,它可以存储任何类型的函数对象。然而,如果要将一个特定类型的函数转换为接受 `std::default_random_engine` 参数并返回 `double` 的 `std::function`,通常需要明确地创建一个适配器或者模板函数来进行这种类型转换。
如果你有一个自定义的函数类型,例如:
```cpp
struct MyCustomFunction {
double operator()(std::default_random_engine &gen) const {
// 函数体
}
};
```
直接将其转换为 `std::function<double(std::default_random_engine&)>` 是不可能的,因为 C++ 编译器不会自动推导这样的转换。你需要手动创建一个转换函数或者一个 `std::bind` 来包装你的函数,使其匹配目标类型。例如:
```cpp
std::function<double(std::default_random_engine&)> wrappedFunc = std::bind(&MyCustomFunction::operator(), myCustomObject, std::placeholders::_1);
```
这里 `myCustomObject` 是 `MyCustomFunction` 类的一个实例。
阅读全文
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
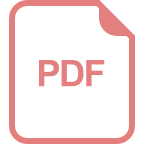
C++标准库:std_set作为一个有序集合
3. 查找元素:使用find函数可以在集合中查找元素,例如`std::set<int>::const_iterator result = set.find(98)`。 4. 交换集合:使用std::swap函数可以交换两个集合的内容,例如`std::swap(set, other)`。 5. 清楚...
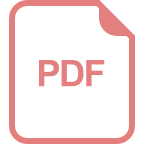
C++ 中boost::share_ptr智能指针的使用方法
std::cout << pTemp->getMember() << std::endl; ``` 这里通过 pTemp 智能指针访问 CTest 对象的成员函数 getMember()。 5. 让智能指针为空 可以使用 reset() 函数让智能指针为空,例如: ```cpp pTemp.reset(); `...
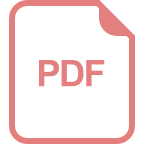
C++11新特性std::tuple的使用方法
double score = std::get<0>(t1); std::string name = std::get<1>(t1); ``` 7. **元组元素的比较**:`std::tuple`支持比较操作,如`==`, `!=`, `<`, `<=`, `>`, `>=`,这些操作符会按照元素的顺序逐个比较。 8...
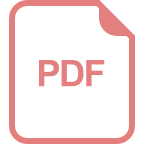
基于双区间熵重映射的图像对比度增强方法研究
内容概要:该论文介绍了一种基于双区间熵重映射的图像对比度增强方法。文中详细解释了方法的数学原理及其在图像处理中的应用,特别是如何有效地提高低对比度图像的对比度,同时对高对比度图像的变换则相对平滑。实验证明该方法不仅提高了图像的视觉效果,还能结合Gabor滤波器进一步提升增强效果。
适合人群:从事图像处理和计算机视觉领域的研究人员和技术人员,以及对图像对比度增强技术感兴趣的学术界人士。
使用场景及目标:适用于需要提高数字图像对比度的各种应用场景,尤其是在图像处理和计算机视觉任务中。目的是提高图像质量,更好地识别和分析图像内容。
其他说明:该方法不仅在对比度增强方面表现优异,还在保持图像原有细节和减少噪声方面显示出优势。通过对多种经典测试图像的评估,证明了其优越性和普适性。
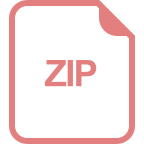
构建基于Django和Stripe的SaaS应用教程
资源摘要信息: "本资源是一套使用Django框架开发的SaaS应用程序,集成了Stripe支付处理和Neon PostgreSQL数据库,前端使用了TailwindCSS进行设计,并通过GitHub Actions进行自动化部署和管理。"
知识点概述:
1. Django框架: Django是一个高级的Python Web框架,它鼓励快速开发和干净、实用的设计。它是一个开源的项目,由经验丰富的开发者社区维护,遵循“不要重复自己”(DRY)的原则。Django自带了一个ORM(对象关系映射),可以让你使用Python编写数据库查询,而无需编写SQL代码。
2. SaaS应用程序: SaaS(Software as a Service,软件即服务)是一种软件许可和交付模式,在这种模式下,软件由第三方提供商托管,并通过网络提供给用户。用户无需将软件安装在本地电脑上,可以直接通过网络访问并使用这些软件服务。
3. Stripe支付处理: Stripe是一个全面的支付平台,允许企业和个人在线接收支付。它提供了一套全面的API,允许开发者集成支付处理功能。Stripe处理包括信用卡支付、ACH转账、Apple Pay和各种其他本地支付方式。
4. Neon PostgreSQL: Neon是一个云原生的PostgreSQL服务,它提供了数据库即服务(DBaaS)的解决方案。Neon使得部署和管理PostgreSQL数据库变得更加容易和灵活。它支持高可用性配置,并提供了自动故障转移和数据备份。
5. TailwindCSS: TailwindCSS是一个实用工具优先的CSS框架,它旨在帮助开发者快速构建可定制的用户界面。它不是一个传统意义上的设计框架,而是一套工具类,允许开发者组合和自定义界面组件而不限制设计。
6. GitHub Actions: GitHub Actions是GitHub推出的一项功能,用于自动化软件开发工作流程。开发者可以在代码仓库中设置工作流程,GitHub将根据代码仓库中的事件(如推送、拉取请求等)自动执行这些工作流程。这使得持续集成和持续部署(CI/CD)变得简单而高效。
7. PostgreSQL: PostgreSQL是一个对象关系数据库管理系统(ORDBMS),它使用SQL作为查询语言。它是开源软件,可以在多种操作系统上运行。PostgreSQL以支持复杂查询、外键、触发器、视图和事务完整性等特性而著称。
8. Git: Git是一个开源的分布式版本控制系统,用于敏捷高效地处理任何或小或大的项目。Git由Linus Torvalds创建,旨在快速高效地处理从小型到大型项目的所有内容。Git是Django项目管理的基石,用于代码版本控制和协作开发。
通过上述知识点的结合,我们可以构建出一个具备现代Web应用程序所需所有关键特性的SaaS应用程序。Django作为后端框架负责处理业务逻辑和数据库交互,而Neon PostgreSQL提供稳定且易于管理的数据库服务。Stripe集成允许处理多种支付方式,使用户能够安全地进行交易。前端使用TailwindCSS进行快速设计,同时GitHub Actions帮助自动化部署流程,确保每次代码更新都能够顺利且快速地部署到生产环境。整体来看,这套资源涵盖了从前端到后端,再到部署和支付处理的完整链条,是构建现代SaaS应用的一套完整解决方案。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
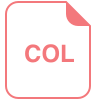
R语言数据处理与GoogleVIS集成:一步步教你绘图
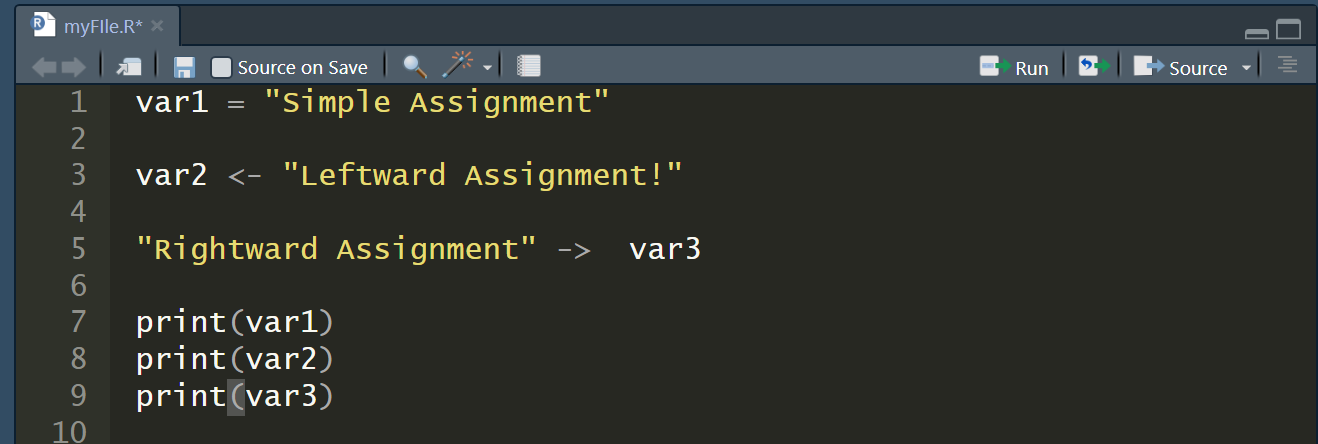
# 1. R语言数据处理基础
在数据分析领域,R语言凭借其强大的统计分析能力和灵活的数据处理功能成为了数据科学家的首选工具。本章将探讨R语言的基本数据处理流程,为后续章节中利用R语言与GoogleVIS集成进行复杂的数据可视化打下坚实的基础。
## 1.1 R语言概述
R语言是一种开源的编程语言,主要用于统计计算和图形表示。它以数据挖掘和分析为核心,拥有庞大的社区支持和丰富的第

如何使用Matlab实现PSO优化SVM进行多输出回归预测?请提供基本流程和关键步骤。
在研究机器学习和数据预测领域时,掌握如何利用Matlab实现PSO优化SVM算法进行多输出回归预测,是一个非常实用的技能。为了帮助你更好地掌握这一过程,我们推荐资源《PSO-SVM多输出回归预测与Matlab代码实现》。通过学习此资源,你可以了解到如何使用粒子群算法(PSO)来优化支持向量机(SVM)的参数,以便进行多输入多输出的回归预测。
参考资源链接:[PSO-SVM多输出回归预测与Matlab代码实现](https://wenku.csdn.net/doc/3i8iv7nbuw?spm=1055.2569.3001.10343)
首先,你需要安装Matlab环境,并熟悉其基本操作。接
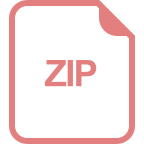
Symfony2框架打造的RESTful问答系统icare-server
资源摘要信息:"icare-server是一个基于Symfony2框架开发的RESTful问答系统。Symfony2是一个使用PHP语言编写的开源框架,遵循MVC(模型-视图-控制器)设计模式。本项目完成于2014年11月18日,标志着其开发周期的结束以及初步的稳定性和可用性。"
Symfony2框架是一个成熟的PHP开发平台,它遵循最佳实践,提供了一套完整的工具和组件,用于构建可靠的、可维护的、可扩展的Web应用程序。Symfony2因其灵活性和可扩展性,成为了开发大型应用程序的首选框架之一。
RESTful API( Representational State Transfer的缩写,即表现层状态转换)是一种软件架构风格,用于构建网络应用程序。这种风格的API适用于资源的表示,符合HTTP协议的方法(GET, POST, PUT, DELETE等),并且能够被多种客户端所使用,包括Web浏览器、移动设备以及桌面应用程序。
在本项目中,icare-server作为一个问答系统,它可能具备以下功能:
1. 用户认证和授权:系统可能支持通过OAuth、JWT(JSON Web Tokens)或其他安全机制来进行用户登录和权限验证。
2. 问题的提交与管理:用户可以提交问题,其他用户或者系统管理员可以对问题进行管理,比如标记、编辑、删除等。
3. 回答的提交与管理:用户可以对问题进行回答,回答可以被其他用户投票、评论或者标记为最佳答案。
4. 分类和搜索:问题和答案可能按类别进行组织,并提供搜索功能,以便用户可以快速找到他们感兴趣的问题。
5. RESTful API接口:系统提供RESTful API,便于开发者可以通过标准的HTTP请求与问答系统进行交互,实现数据的读取、创建、更新和删除操作。
Symfony2框架对于RESTful API的开发提供了许多内置支持,例如:
- 路由(Routing):Symfony2的路由系统允许开发者定义URL模式,并将它们映射到控制器操作上。
- 请求/响应对象:处理HTTP请求和响应流,为开发RESTful服务提供标准的方法。
- 验证组件:可以用来验证传入请求的数据,并确保数据的完整性和正确性。
- 单元测试:Symfony2鼓励使用PHPUnit进行单元测试,确保RESTful服务的稳定性和可靠性。
对于使用PHP语言的开发者来说,icare-server项目的完成和开源意味着他们可以利用Symfony2框架的优势,快速构建一个功能完备的问答系统。通过学习icare-server项目的代码和文档,开发者可以更好地掌握如何构建RESTful API,并进一步提升自身在Web开发领域的专业技能。同时,该项目作为一个开源项目,其代码结构、设计模式和实现细节等都可以作为学习和实践的最佳范例。
由于icare-server项目完成于2014年,使用的技术栈可能不是最新的,因此在考虑实际应用时,开发者可能需要根据当前的技术趋势和安全要求进行相应的升级和优化。例如,PHP的版本更新可能带来新的语言特性和改进的安全措施,而Symfony2框架本身也在不断地发布新版本和更新补丁,因此维护一个长期稳定的问答系统需要开发者对技术保持持续的关注和学习。

"互动学习:行动中的多样性与论文攻读经历"
多样性她- 事实上SCI NCES你的时间表ECOLEDO C Tora SC和NCESPOUR l’Ingén学习互动,互动学习以行动为中心的强化学习学会互动,互动学习,以行动为中心的强化学习计算机科学博士论文于2021年9月28日在Villeneuve d'Asq公开支持马修·瑟林评审团主席法布里斯·勒菲弗尔阿维尼翁大学教授论文指导奥利维尔·皮耶昆谷歌研究教授:智囊团论文联合主任菲利普·普雷教授,大学。里尔/CRISTAL/因里亚报告员奥利维耶·西格德索邦大学报告员卢多维奇·德诺耶教授,Facebook /索邦大学审查员越南圣迈IMT Atlantic高级讲师邀请弗洛里安·斯特鲁布博士,Deepmind对于那些及时看到自己错误的人...3谢谢你首先,我要感谢我的两位博士生导师Olivier和Philippe。奥利维尔,"站在巨人的肩膀上"这句话对你来说完全有意义了。从科学上讲,你知道在这篇论文的(许多)错误中,你是我可以依