用c++实现基于openssl的大文件的签名并将签名写入文件中以及验证签名
时间: 2024-02-27 20:55:05 浏览: 23
好的,我将为您提供一个基于openssl的C++程序,实现对大文件进行数字签名并将签名写入文件中,以及验证签名。
首先,您需要安装openssl库和头文件,以便在您的程序中使用openssl的函数。接下来,您可以使用以下代码实现数字签名:
```
#include <openssl/evp.h>
#include <openssl/sha.h>
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
#define KEY_LENGTH 2048
#define SIGN_LENGTH 256
bool sign_file(const char* file_path, const char* private_key_path, const char* signature_path) {
ifstream file(file_path, ios::binary | ios::ate);
if (!file.is_open()) {
cout << "Failed to open file" << endl;
return false;
}
int file_size = file.tellg();
file.seekg(0, ios::beg);
unsigned char* file_data = new unsigned char[file_size];
file.read((char*)file_data, file_size);
file.close();
EVP_PKEY* pkey = NULL;
FILE* fp = fopen(private_key_path, "rb");
if (!fp) {
cout << "Failed to open private key file" << endl;
return false;
}
pkey = PEM_read_PrivateKey(fp, NULL, NULL, NULL);
fclose(fp);
if (!pkey) {
cout << "Failed to read private key" << endl;
return false;
}
EVP_MD_CTX* mdctx = EVP_MD_CTX_new();
if (!mdctx) {
cout << "Failed to create md context" << endl;
return false;
}
if (!EVP_SignInit(mdctx, EVP_sha256())) {
cout << "Failed to initialize signing" << endl;
return false;
}
if (!EVP_SignUpdate(mdctx, file_data, file_size)) {
cout << "Failed to update signing" << endl;
return false;
}
unsigned char* signature = new unsigned char[SIGN_LENGTH];
unsigned int signature_length = SIGN_LENGTH;
if (!EVP_SignFinal(mdctx, signature, &signature_length, pkey)) {
cout << "Failed to finalize signing" << endl;
return false;
}
ofstream signature_file(signature_path, ios::binary | ios::trunc);
if (!signature_file.is_open()) {
cout << "Failed to create signature file" << endl;
return false;
}
signature_file.write((char*)signature, signature_length);
signature_file.close();
delete[] file_data;
delete[] signature;
EVP_MD_CTX_free(mdctx);
EVP_PKEY_free(pkey);
return true;
}
```
此函数将读取指定的文件,并使用提供的私钥对其进行数字签名。签名将写入指定的文件中。请注意,此函数使用SHA256哈希算法进行加密。您可以根据需要更改哈希算法。
接下来,您可以使用以下代码验证签名:
```
bool verify_signature(const char* file_path, const char* public_key_path, const char* signature_path) {
ifstream file(file_path, ios::binary | ios::ate);
if (!file.is_open()) {
cout << "Failed to open file" << endl;
return false;
}
int file_size = file.tellg();
file.seekg(0, ios::beg);
unsigned char* file_data = new unsigned char[file_size];
file.read((char*)file_data, file_size);
file.close();
EVP_PKEY* pkey = NULL;
FILE* fp = fopen(public_key_path, "rb");
if (!fp) {
cout << "Failed to open public key file" << endl;
return false;
}
pkey = PEM_read_PUBKEY(fp, NULL, NULL, NULL);
fclose(fp);
if (!pkey) {
cout << "Failed to read public key" << endl;
return false;
}
ifstream signature_file(signature_path, ios::binary | ios::ate);
if (!signature_file.is_open()) {
cout << "Failed to open signature file" << endl;
return false;
}
int signature_size = signature_file.tellg();
signature_file.seekg(0, ios::beg);
unsigned char* signature = new unsigned char[signature_size];
signature_file.read((char*)signature, signature_size);
signature_file.close();
EVP_MD_CTX* mdctx = EVP_MD_CTX_new();
if (!mdctx) {
cout << "Failed to create md context" << endl;
return false;
}
if (!EVP_VerifyInit(mdctx, EVP_sha256())) {
cout << "Failed to initialize verification" << endl;
return false;
}
if (!EVP_VerifyUpdate(mdctx, file_data, file_size)) {
cout << "Failed to update verification" << endl;
return false;
}
int result = EVP_VerifyFinal(mdctx, signature, signature_size, pkey);
if (result != 1) {
cout << "Failed to verify signature" << endl;
return false;
}
delete[] file_data;
delete[] signature;
EVP_MD_CTX_free(mdctx);
EVP_PKEY_free(pkey);
return true;
}
```
此函数将读取指定的文件和签名文件,并使用提供的公钥验证签名是否有效。如果签名有效,则返回true,否则返回false。
请注意,此程序中使用的密钥长度为2048位。您可以根据需要更改密钥长度。此外,此程序中使用的哈希算法为SHA256。您可以根据需要更改哈希算法。
希望这能帮到您!
相关推荐
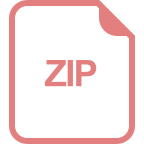
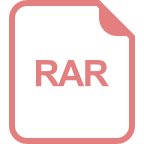














