(1). 在应用程序窗体中安排两个文本框分别用来输入两个整数,两个按钮分别为“+”, “*”,一个结果标签。点击按纽“+”将两文本框的数据做加法运算;点击按钮“*”做 乘法运算,将结果显示在标签中,完成下面未完成的程序并要求给整体代码加上注释。 Input: import java.awt.event.*; import java.math.BigInteger; import java.awt.*; import javax.swing.*; public class Caculate1 extends JFrame{ JTextArea text1,text2; JButton jb1,jb2; JLabel label; Caculate1(){ text1=new JTextArea(2,4); text2=new JTextArea(2,4); jb1=new JButton("+"); jb2=new JButton("*"); label=new JLabel(); JSplitPane p1; JPanel p2=new JPanel(); jb1.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { String n1=text1.getText(); String n2=text2.getText(); try { BigInteger num1=new BigInteger(n1); BigInteger num2=new BigInteger(n2); label.setText((num1.add(num2)).toString()); }catch (Exception ee) { label.setText("请输入整数"); text1.setText(null); text2.setText(null); } } }); jb2.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { String n1=text1.getText(); String n2=text2.getText(); try { BigInteger num1=new BigInteger(n1); BigInteger num2=new BigInteger(n2); label.setText((num1.multiply(num2)).toString()); }catch (Exception ee) { label.setText("请输入整数"); text1.setText(null); text2.setText(null); } } }); setLayout(new GridLayout(3,1)); p1=new JSplitPane(JSplitPane.HORIZONTAL_SPLIT,text1,text2); p1.setDividerLocation(117); add(p1); p2.setLayout(new GridLayout(1,2)); p2.add(jb1); p2.add(jb2); add(p2); add(label); setBounds(100,100,260,190); setVisible(true); validate(); setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE); }
时间: 2024-01-12 13:03:41 浏览: 42
好的,这是一个 Java 的应用程序窗体,我来给您加上注释:
```java
import java.awt.event.*;
import java.math.BigInteger;
import java.awt.*;
import javax.swing.*;
public class Caculate1 extends JFrame{
JTextArea text1,text2;
JButton jb1,jb2;
JLabel label;
Caculate1(){
// 创建两个文本框,用来输入两个整数
text1=new JTextArea(2,4);
text2=new JTextArea(2,4);
// 创建两个按钮,分别为“+”和“*”
jb1=new JButton("+");
jb2=new JButton("*");
// 创建结果标签
label=new JLabel();
JSplitPane p1; // 用来创建一个分割窗格
JPanel p2=new JPanel(); // 创建一个面板
// 给“+”按钮绑定事件
jb1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String n1=text1.getText(); // 获取第一个文本框的数据
String n2=text2.getText(); // 获取第二个文本框的数据
try {
BigInteger num1=new BigInteger(n1); // 将第一个数据转为 BigInteger 类型
BigInteger num2=new BigInteger(n2); // 将第二个数据转为 BigInteger 类型
label.setText((num1.add(num2)).toString()); // 将两个数据相加,并将结果显示在标签中
}catch (Exception ee) {
label.setText("请输入整数"); // 如果用户输入的数据不是整数,则在标签中显示错误信息
text1.setText(null); // 清空第一个文本框
text2.setText(null); // 清空第二个文本框
}
}
});
// 给“*”按钮绑定事件
jb2.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String n1=text1.getText(); // 获取第一个文本框的数据
String n2=text2.getText(); // 获取第二个文本框的数据
try {
BigInteger num1=new BigInteger(n1); // 将第一个数据转为 BigInteger 类型
BigInteger num2=new BigInteger(n2); // 将第二个数据转为 BigInteger 类型
label.setText((num1.multiply(num2)).toString()); // 将两个数据相乘,并将结果显示在标签中
}catch (Exception ee) {
label.setText("请输入整数"); // 如果用户输入的数据不是整数,则在标签中显示错误信息
text1.setText(null); // 清空第一个文本框
text2.setText(null); // 清空第二个文本框
}
}
});
setLayout(new GridLayout(3,1)); // 设置布局为3行1列的网格布局
p1=new JSplitPane(JSplitPane.HORIZONTAL_SPLIT,text1,text2); // 创建一个水平方向的分割窗格,将两个文本框放入其中
p1.setDividerLocation(117); // 设置分割窗格的位置
add(p1); // 将分割窗格添加到主窗口中
p2.setLayout(new GridLayout(1,2)); // 设置面板布局为1行2列的网格布局
p2.add(jb1); // 将“+”按钮添加到面板中
p2.add(jb2); // 将“*”按钮添加到面板中
add(p2); // 将面板添加到主窗口中
add(label); // 将结果标签添加到主窗口中
setBounds(100,100,260,190); // 设置主窗口的位置和大小
setVisible(true); // 将主窗口设置为可见
validate(); // 验证主窗口
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE); // 设置关闭窗口时的操作为销毁窗口
}
}
```
相关推荐
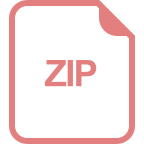
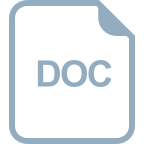
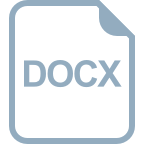















