用ASP.NET Mvc 编写一个汽车管理系统
时间: 2023-08-20 16:03:57 浏览: 94
好的,下面是汽车管理系统的简单实现:
1. 创建一个 ASP.NET Mvc 项目并命名为 CarManagementSystem。
2. 在 Models 文件夹下创建一个名为 Car.cs 的类,定义汽车的属性:
```csharp
public class Car
{
public int Id { get; set; }
public string Brand { get; set; }
public string Model { get; set; }
public int Year { get; set; }
public double Price { get; set; }
}
```
3. 在 Controllers 文件夹下创建一个名为 CarController.cs 的控制器,定义汽车管理系统的操作:
```csharp
public class CarController : Controller
{
private List<Car> cars = new List<Car>()
{
new Car { Id = 1, Brand = "Toyota", Model = "Camry", Year = 2018, Price = 25000 },
new Car { Id = 2, Brand = "Honda", Model = "Accord", Year = 2017, Price = 22000 },
new Car { Id = 3, Brand = "Ford", Model = "Mustang", Year = 2019, Price = 35000 },
new Car { Id = 4, Brand = "Chevrolet", Model = "Camaro", Year = 2016, Price = 30000 },
new Car { Id = 5, Brand = "Nissan", Model = "Altima", Year = 2017, Price = 20000 }
};
// 显示所有汽车信息
public ActionResult Index()
{
return View(cars);
}
// 显示指定汽车信息
public ActionResult Details(int id)
{
var car = cars.FirstOrDefault(c => c.Id == id);
if (car == null)
{
return HttpNotFound();
}
return View(car);
}
// 添加汽车信息
[HttpGet]
public ActionResult Create()
{
return View();
}
[HttpPost]
public ActionResult Create(Car car)
{
if (ModelState.IsValid)
{
car.Id = cars.Max(c => c.Id) + 1;
cars.Add(car);
return RedirectToAction("Index");
}
return View(car);
}
// 修改汽车信息
[HttpGet]
public ActionResult Edit(int id)
{
var car = cars.FirstOrDefault(c => c.Id == id);
if (car == null)
{
return HttpNotFound();
}
return View(car);
}
[HttpPost]
public ActionResult Edit(Car car)
{
if (ModelState.IsValid)
{
var existingCar = cars.FirstOrDefault(c => c.Id == car.Id);
if (existingCar != null)
{
existingCar.Brand = car.Brand;
existingCar.Model = car.Model;
existingCar.Year = car.Year;
existingCar.Price = car.Price;
return RedirectToAction("Index");
}
}
return View(car);
}
// 删除汽车信息
[HttpGet]
public ActionResult Delete(int id)
{
var car = cars.FirstOrDefault(c => c.Id == id);
if (car == null)
{
return HttpNotFound();
}
return View(car);
}
[HttpPost, ActionName("Delete")]
public ActionResult DeleteConfirmed(int id)
{
var car = cars.FirstOrDefault(c => c.Id == id);
if (car == null)
{
return HttpNotFound();
}
cars.Remove(car);
return RedirectToAction("Index");
}
}
```
4. 在 Views 文件夹下创建一个名为 Car 文件夹,并在其中创建以下视图文件:
- Index.cshtml:显示所有汽车信息。
```html
@model List<Car>
<h2>Cars</h2>
<p>
@Html.ActionLink("Add Car", "Create")
</p>
<table class="table">
<tr>
<th>Brand</th>
<th>Model</th>
<th>Year</th>
<th>Price</th>
<th></th>
</tr>
@foreach (var car in Model)
{
<tr>
<td>@car.Brand</td>
<td>@car.Model</td>
<td>@car.Year</td>
<td>@car.Price.ToString("C")</td>
<td>
@Html.ActionLink("Details", "Details", new { id = car.Id }) |
@Html.ActionLink("Edit", "Edit", new { id = car.Id }) |
@Html.ActionLink("Delete", "Delete", new { id = car.Id }, new { onclick = "return confirm('Are you sure you want to delete this car?')" })
</td>
</tr>
}
</table>
```
- Details.cshtml:显示指定汽车信息。
```html
@model Car
<h2>@Model.Brand @Model.Model</h2>
<dl class="dl-horizontal">
<dt>Year</dt>
<dd>@Model.Year</dd>
<dt>Price</dt>
<dd>@Model.Price.ToString("C")</dd>
</dl>
<p>
@Html.ActionLink("Edit", "Edit", new { id = Model.Id }) |
@Html.ActionLink("Back to List", "Index")
</p>
```
- Create.cshtml:添加汽车信息。
```html
@model Car
<h2>Add Car</h2>
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<hr />
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
<div class="form-group">
@Html.LabelFor(model => model.Brand, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Brand, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Brand, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Model, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Model, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Model, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Year, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Year, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Year, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Price, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Price, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Price, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Save" class="btn btn-default" />
</div>
</div>
</div>
}
<div>
@Html.ActionLink("Back to List", "Index")
</div>
```
- Edit.cshtml:修改汽车信息。
```html
@model Car
<h2>Edit Car</h2>
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<hr />
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
@Html.HiddenFor(model => model.Id)
<div class="form-group">
@Html.LabelFor(model => model.Brand, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Brand, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Brand, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Model, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Model, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Model, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Year, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Year, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Year, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Price, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Price, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Price, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Save" class="btn btn-default" />
</div>
</div>
</div>
}
<div>
@Html.ActionLink("Back to List", "Index")
</div>
```
- Delete.cshtml:删除汽车信息。
```html
@model Car
<h2>Delete Car</h2>
<h3>Are you sure you want to delete this car?</h3>
<dl class="dl-horizontal">
<dt>Brand</dt>
<dd>@Model.Brand</dd>
<dt>Model</dt>
<dd>@Model.Model</dd>
<dt>Year</dt>
<dd>@Model.Year</dd>
<dt>Price</dt>
<dd>@Model.Price.ToString("C")</dd>
</dl>
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Delete" class="btn btn-default" />
</div>
</div>
}
<div>
@Html.ActionLink("Back to List", "Index")
</div>
```
5. 运行项目,即可看到汽车管理系统的界面。
相关推荐
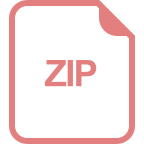
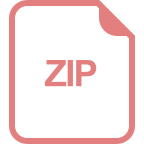
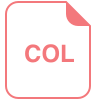
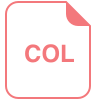
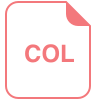
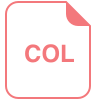
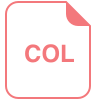









