from pytmx.util_pygame import load_pygame
时间: 2024-04-21 21:20:03 浏览: 205
`from pytmx.util_pygame import load_pygame` 是一个Python代码行,它导入了`pytmx.util_pygame`模块中的`load_pygame`函数。这个函数用于加载和解析TMX(Tiled Map XML)格式的地图文件,并将其转换为Pygame游戏引擎可用的数据结构。
TMX是一种常用的地图编辑器文件格式,它可以描述游戏中的地图、瓦片集合、碰撞区域等信息。`load_pygame`函数可以帮助我们将TMX文件加载到Pygame中,以便在游戏中使用。
如果你想使用`load_pygame`函数,你需要先安装`pytmx`库。你可以使用以下命令来安装它:
```
pip install pytmx
```
然后,在你的Python代码中,你可以使用`from pytmx.util_pygame import load_pygame`来导入并使用`load_pygame`函数。
相关问题
module 'pytmx' has no attribute 'load_pygame'怎么解决
当你遇到 "module 'pytmx' has no attribute 'load_pygame'" 这样的错误时,通常意味着你在尝试使用 `pytmx` 库中的 `load_pygame` 方法,但这个方法在你当前的 `pytmx` 版本中可能不存在或已被移除。`pytmx` 是一个用于加载和处理Tiled地图的数据格式的库,它可能在不同版本中功能有所变化。
要解决这个问题,你可以尝试以下几个步骤:
1. **更新库版本**:确保你使用的 `pytmx` 版本支持 `load_pygame` 功能。如果版本过旧,你可能需要升级到最新版。
2. **查看文档**:查阅 `pytmx` 最新文档,确认 `load_pygame` 是否还在官方推荐的用法范围内,或者是否已被替换为其他方法。
3. **代码检查**:确保你没有误写或拼写错误,检查导入语句是否正确,比如 `from pytmx.load_pygame import load` 或者 `from pytmx import load_pygame`。
4. **错误提示**:如果安装了多个 `pytmx` 版本,检查你的项目可能包含了多个版本,这可能导致版本冲突。尝试清除虚拟环境或Python安装目录下的缓存,然后重新安装。
5. **报错日志**:如果上述方法无效,检查错误堆栈,看是否有更详细的错误信息,这可能提供关于问题根源的线索。
load_tilemap():读取tiled绘制的地图信息 Scene.from_tilemap:将地图信息提取到Scene对象中
在游戏开发中,tiled地图编辑器是一个非常流行的工具,用于创建复杂的2D地图。使用Python和Pygame等库加载和显示tiled绘制的地图信息,可以大大简化开发过程。以下是`load_tilemap()`和`Scene.from_tilemap`的使用方法:
### `load_tilemap()`
`load_tilemap()`函数用于读取tiled绘制的地图信息。这个函数通常会解析tiled生成的TMX文件,并将地图的各个图层、对象和属性提取出来。
```python
def load_tilemap(file_path):
import pytmx
from pytmx.util_pygame import load_pygame
# 加载tiled地图
tmx_data = load_pygame(file_path)
# 提取地图信息
map_width = tmx_data.width
map_height = tmx_data.height
tile_width = tmx_data.tilewidth
tile_height = tmx_data.tileheight
# 提取图层信息
layers = []
for layer in tmx_data.visible_layers:
if isinstance(layer, pytmx.TiledTileLayer):
layer_data = []
for x, y, gid in layer:
layer_data.append(gid)
layers.append(layer_data)
# 提取对象信息
objects = []
for obj in tmx_data.objects:
obj_data = {
'name': obj.name,
'x': obj.x,
'y': obj.y,
'width': obj.width,
'height': obj.height,
'properties': obj.properties
}
objects.append(obj_data)
return {
'width': map_width,
'height': map_height,
'tile_width': tile_width,
'tile_height': tile_height,
'layers': layers,
'objects': objects
}
```
### `Scene.from_tilemap`
`Scene.from_tilemap`方法用于将地图信息提取到`Scene`对象中。这个方法通常会创建一个`Scene`对象,并将地图的图层和对象添加到场景中。
```python
class Scene:
def __init__(self):
self.layers = []
self.objects = []
@classmethod
def from_tilemap(cls, tilemap_data):
scene = cls()
scene.width = tilemap_data['width']
scene.height = tilemap_data['height']
scene.tile_width = tilemap_data['tile_width']
scene.tile_height = tilemap_data['tile_height']
# 添加图层
for layer_data in tilemap_data['layers']:
layer = Layer(layer_data)
scene.layers.append(layer)
# 添加对象
for obj_data in tilemap_data['objects']:
obj = GameObject(obj_data)
scene.objects.append(obj)
return scene
class Layer:
def __init__(self, data):
self.data = data
class GameObject:
def __init__(self, data):
self.name = data['name']
self.x = data['x']
self.y = data['y']
self.width = data['width']
self.height = data['height']
self.properties = data['properties']
```
### 使用示例
```python
tilemap_file = 'path/to/your/tilemap.tmx'
tilemap_data = load_tilemap(tilemap_file)
scene = Scene.from_tilemap(tilemap_data)
# 现在你可以使用scene对象来渲染地图和处理游戏逻辑
```
阅读全文
相关推荐


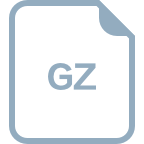



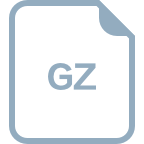







