基于sklearn的cluster包中提供的方法实现kmeans聚类算法,完整源码
时间: 2024-09-30 17:10:59 浏览: 20
基于Scikit-learn库的KMeans聚类算法是一种无监督学习方法,主要用于将数据集划分为若干个簇(clusters)。以下是使用Scikit-learn的`KMeans`类来实现K-means的基本步骤和部分源码:
```python
from sklearn.cluster import KMeans
import numpy as np
# 创建KMeans实例
kmeans = KMeans(n_clusters=3) # 选择3个簇
# 假设你有一个二维numpy数组作为输入数据
data = np.array([[5, 8], [1, 2], [9, 4], [4, 7], [6, 6], [3, 3]]) # 示例数据
# 使用fit()方法训练模型
kmeans.fit(data)
# 获取每个样本所属的簇标签
labels = kmeans.labels_
# 可视化结果,如果使用的是matplotlib,可以这样做
centroids = kmeans.cluster_centers_
plt.scatter(data[:, 0], data[:, 1], c=labels, cmap='viridis')
plt.scatter(centroids[:, 0], centroids[:, 1], marker='x', s=100, linewidths=3, color='red')
#
相关问题
基于sklearn的cluster包中提供的方法实现kmeans聚类算法。
在scikit-learn库中,K-means聚类是一种常用的无监督学习方法,用于将数据集划分为预设数量的类别。以下是基于`sklearn.cluster.KMeans`实现K-means聚类的基本步骤:
1. **导入必要的库**:
```python
from sklearn.cluster import KMeans
import numpy as np
```
2. **加载或准备数据**:
确保数据是数值型并且已经归一化或标准化,因为K-means对输入敏感。
3. **创建KMeans实例并设置参数**:
```python
n_clusters = 3 # 要确定的簇的数量
kmeans = KMeans(n_clusters=n_clusters)
```
4. **拟合数据**:
```python
X = ... # 你的数据,例如二维数组
kmeans.fit(X)
```
5. **获取聚类结果**:
```python
labels = kmeans.labels_ # 获取每个样本所属的簇标签
centroids = kmeans.cluster_centers_ # 获取各个簇的质心
```
6. **可视化结果** (如果数据维度低):
```python
import matplotlib.pyplot as plt
plt.scatter(X[:,0], X[:,1], c=labels, cmap='viridis')
plt.scatter(centroids[:,0], centroids[:,1], color='red', marker='x')
plt.show()
```
sklearn的cluster包中提供的方法实现kmeans聚类算法
sklearn库中的`cluster`模块提供了KMeans算法用于数据聚类,这是基于密度的无监督学习方法。K-Means的主要工作原理如下:
1. **初始化中心点**:随机选择k个数据点作为初始质心(中心点)。
2. **分配样本到最近的中心**:每个样本被分配到距离它最近的质心所属的簇。
3. **更新中心点**:计算每个簇内的所有样本的均值,将这个均值作为新的质心。
4. **迭代直到收敛**:重复步骤2和3,直到簇的分配不再改变或达到预设的最大迭代次数。
`sklearn.cluster.KMeans`类实现了这一过程。关键函数包括:
- `fit(X)`:对输入数据X进行聚类,返回聚类标签。
- `fit_predict(X)`:同时进行训练并返回聚类标签。
- `predict(X)`:对未训练过的数据进行预测,返回最接近的簇的标签。
要使用该算法,你需要先导入`KMeans`,然后实例化模型并调用相应方法:
```python
from sklearn.cluster import KMeans
# 初始化模型,例如设定k=3
kmeans = KMeans(n_clusters=3)
# 使用数据拟合模型
kmeans.fit(X_train)
# 预测新数据的聚类
labels = kmeans.predict(X_test)
```
相关推荐
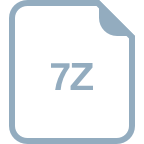
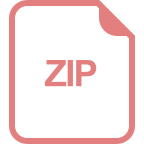
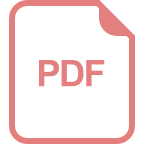












