用C语言写出学生数据元素(学号1,2,3…10,姓名) 10个 对数据元素进行查找、删除5、插入5 分别采用顺序和链式进行存储的算法
时间: 2024-09-15 20:11:33 浏览: 28
在C语言中,我们可以分别用顺序表(数组)和链表结构来实现对学生数据元素的存储、查找、插入和删除操作。
### 1. 顺序存储 (数组)
```c
typedef struct {
int student_id;
char name[20];
} Student;
// 顺序存储结构
Student students[10];
// 插入函数(假设索引从0开始)
void insert_student(int index, const char* name) {
if (index >= 10) return; // 如果超过数组大小
students[index].student_id = index + 1; // 假设学生编号递增
strcpy(students[index].name, name);
}
// 删除第5个学生
void delete_student() {
if (5 < 0 || 5 >= 10) return;
memmove(&students[5], &students[6], sizeof(Student) * (9 - 5)); // 移动元素
students[9] = (Student){0, ""}; // 清空最后一个位置
}
// 查找指定学生
int find_student(const char* name) {
for (int i = 0; i < 10; ++i) {
if (strcmp(students[i].name, name) == 0) return i + 1; // 返回学生编号
}
return -1; // 未找到
}
```
### 2. 链表存储 (动态分配)
```c
typedef struct Node {
int student_id;
char name[20];
struct Node* next;
} Node, *StudentList;
// 创建链表头节点
Node* head = NULL;
// 插入函数
void insert_student(Node** head, const char* name) {
Node* newNode = malloc(sizeof(Node));
newNode->student_id = ++(*head)->count;
strcpy(newNode->name, name);
newNode->next = (*head);
(*head) = newNode;
}
// 删除第5个学生(这里需要遍历链表)
void delete_student(Node** head) {
if (!(*head)) return;
if ((*head)->count == 5) { // 如果待删的是第一个节点
Node* temp = *head;
*head = (*head)->next;
free(temp);
} else {
Node* current = *head;
while (current->next && current->next->count != 5) {
current = current->next;
}
if (current->next) {
Node* temp = current->next;
current->next = current->next->next;
free(temp);
}
}
}
// 查找指定学生
Node* find_student(char* name) {
Node* current = head;
while (current) {
if (strcmp(current->name, name) == 0) return current;
current = current->next;
}
return NULL;
}
```
**相关问题--:**
1. 顺序存储和链表存储相比,哪一种更适合频繁插入和删除操作?
2. 这两种方式如何处理数据过多的情况?
3. 如果我想在链表中添加一个搜索功能,应该怎么做?
相关推荐
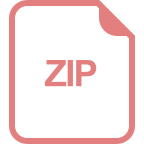
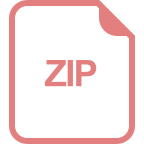














