创建一个date类型日期,包含year,month,day
时间: 2024-10-19 14:13:47 浏览: 35
在编程中,创建一个表示日期的数据结构通常涉及到使用特定语言的内置日期或日期时间库。例如,在JavaScript中,你可以使用`Date`对象来表示日期,它的构造函数需要年、月和日作为参数:
```javascript
let date = new Date();
date.setFullYear(2023); // 设置年份
date.setMonth(0); // 设置月份(注意0代表 January)
date.setDate(1); // 设置日期(默认第一天)
console.log(date); // 输出类似 "Tue Jan 01 2023 00:00:00 GMT+0800 (China Standard Time)"
```
如果你需要一个更具灵活性的对象模型,可以自定义一个类来封装这些属性:
```javascript
class CustomDate {
constructor(year, month, day) {
this.year = year;
this.month = month;
this.day = day;
}
}
const customDate = new CustomDate(2023, 1, 1);
```
在这个例子中,`CustomDate`是一个简单的日期类,允许你指定具体的年、月和日。
相关问题
C语言计算一个日期是当年中的第几天,定义一个结构体类型Date,包括整型成员year、month、day 。定义一个Date类型变量,注意闰年问题
好的,我可以为您解答此问题。以下是计算一个日期是当年中的第几天的 C 代码:
```
#include <stdio.h>
struct Date {
int year;
int month;
int day;
};
int isLeapYear(int year) {
return ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0);
}
int dayOfYear(struct Date date) {
int daysInMonth[] = {0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
int day = date.day;
for (int i = 1; i < date.month; ++i) {
day += daysInMonth[i];
}
if (date.month > 2 && isLeapYear(date.year)) {
++day;
}
return day;
}
int main() {
struct Date date = {2021, 7, 15};
int day = dayOfYear(date);
printf("%d-%d-%d is day %d of the year.\n", date.year, date.month, date.day, day);
return 0;
}
```
其中,`isLeapYear` 函数用于判断是否是闰年,如果是则返回 1,否则返回 0。`dayOfYear` 函数用于计算一个日期是当年中的第几天,先根据月份累加天数,然后如果是闰年且月份大于 2 月,则再加 1 天。最后在 `main` 函数中,我们定义一个 `Date` 类型的变量 `date`,并将其初始化为 `{2021, 7, 15}`,即表示 2021 年 7 月 15 日,然后调用 `dayOfYear` 函数计算当年中的第几天,并输出结果。
希望我的回答能够帮到您。
多文件实现定义一个Date类,包括year 、month、day三个数据成员,通过set_date成员函数输入出生日期数据,通过disp_date成员函数将其输出,输出格式如下:
好的,我可以帮你解答这个问题。
首先,我们在一个头文件 `date.h` 中定义 `Date` 类。代码如下:
```cpp
#ifndef DATE_H
#define DATE_H
class Date {
public:
Date(); // 默认构造函数
Date(int year, int month, int day); // 带参数构造函数
void set_date(int year, int month, int day); // 设置出生日期
void disp_date(); // 输出出生日期
private:
int year_; // 年份
int month_; // 月份
int day_; // 日份
};
#endif
```
其中,我们定义了默认构造函数和带参数构造函数,以及 `set_date` 和 `disp_date` 成员函数,用于输入和输出出生日期。
接下来,在一个源文件 `date.cpp` 中实现 `Date` 类的成员函数。代码如下:
```cpp
#include "date.h"
#include <iostream>
using namespace std;
Date::Date() {
year_ = 0;
month_ = 0;
day_ = 0;
}
Date::Date(int year, int month, int day) {
year_ = year;
month_ = month;
day_ = day;
}
void Date::set_date(int year, int month, int day) {
year_ = year;
month_ = month;
day_ = day;
}
void Date::disp_date() {
cout << "出生日期:" << year_ << "年" << month_ << "月" << day_ << "日" << endl;
}
```
最后,在主函数中使用 `Date` 类。代码如下:
```cpp
#include "date.h"
int main() {
Date date;
date.set_date(2000, 1, 1);
date.disp_date();
return 0;
}
```
输出结果如下:
```
出生日期:2000年1月1日
```
这样就实现了定义一个 `Date` 类,并通过多文件实现输入和输出出生日期的功能。
阅读全文
相关推荐












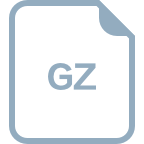


