jsonarray按时间排序
时间: 2023-09-05 14:03:49 浏览: 110
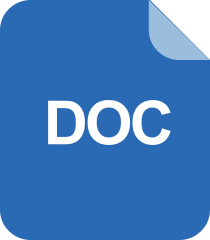
(时间)排序函数、按某一属性排序
要对一个JSONArray按照时间进行排序,可以按照如下步骤进行:
1. 将JSONArray中的元素转换为JSONObject对象,并提取出时间字段的值。
2. 创建一个新的JSONArray用于存储排序后的结果。
3. 使用Collections类的sort()方法来对JSONObject对象按照时间字段进行排序。在sort()方法中自定义比较器Comparator,根据时间字段的值来进行比较。
4. 遍历排序后的JSONObject对象,将其转换为原始的JSON格式,并添加到新的JSONArray中。
5. 最后得到的新的JSONArray即为按时间排序后的结果。
以下是一个示例代码:
```java
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Collections;
import java.util.Comparator;
import java.util.Date;
public class JsonArraySortByTime {
public static void main(String[] args) {
JSONArray jsonArray = new JSONArray();
// 假设jsonArray中的元素为JSONObject对象,包含"time"字段。
jsonArray.put(createJsonObject("2022-01-01 10:00:00"));
jsonArray.put(createJsonObject("2022-01-03 08:00:00"));
jsonArray.put(createJsonObject("2022-01-02 12:00:00"));
JSONArray sortedArray = sortByTime(jsonArray);
System.out.println(sortedArray.toString());
}
private static JSONObject createJsonObject(String time) {
JSONObject jsonObject = new JSONObject();
try {
jsonObject.put("time", time);
} catch (JSONException e) {
e.printStackTrace();
}
return jsonObject;
}
private static JSONArray sortByTime(JSONArray jsonArray) {
JSONArray sortedArray = new JSONArray();
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
try {
// 将JSONArray中的元素转换为JSONObject对象,并提取出时间字段的值。
for (int i = 0; i < jsonArray.length(); i++) {
JSONObject jsonObject = jsonArray.getJSONObject(i);
String timeStr = jsonObject.getString("time");
Date time = format.parse(timeStr);
jsonObject.put("time", time);
sortedArray.put(jsonObject);
}
// 使用Collections类的sort()方法对JSONObject对象按照时间字段进行排序,根据自定义比较器Comparator进行比较。
Collections.sort(sortedArray, new Comparator<Object>() {
public int compare(Object o1, Object o2) {
try {
Date time1 = (Date) ((JSONObject) o1).get("time");
Date time2 = (Date) ((JSONObject) o2).get("time");
return time1.compareTo(time2);
} catch (JSONException e) {
e.printStackTrace();
}
return 0;
}
});
// 遍历排序后的JSONObject对象,转换为原始的JSON格式,并添加到新的JSONArray中。
for (int i = 0; i < sortedArray.length(); i++) {
JSONObject jsonObject = sortedArray.getJSONObject(i);
String timeStr = format.format(jsonObject.get("time"));
jsonObject.put("time", timeStr);
sortedArray.put(i, jsonObject);
}
} catch (JSONException | ParseException e) {
e.printStackTrace();
}
return sortedArray;
}
}
```
上述代码会输出按时间排序后的JSONArray的字符串形式。
阅读全文
相关推荐

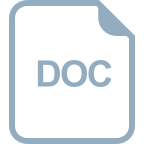



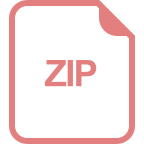
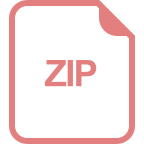
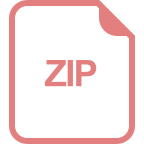