使用c#编写一个类,要求其中包含一个排序的方法sort,如果传入的是一串整数,就按升序排序后输出,如果传入一个字符串,就按字符的降序排序后输出
时间: 2024-10-21 15:17:38 浏览: 26
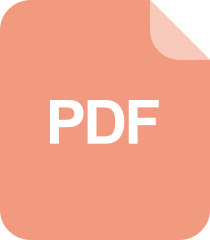
C#通过IComparable实现ListT.sort()排序
在 C# 中,你可以创建一个名为 `Sortable` 的通用类,它有一个泛型方法 `Sort` 来处理整数和字符串的排序需求。这里是一个简单的实现示例:
```csharp
using System;
using System.Collections.Generic;
public class Sortable<T>
{
public void Sort(T[] items)
{
if (items == null || items.Length == 0)
return;
Type elementType = typeof(T);
if (elementType.IsGenericType && elementType.GetGenericTypeDefinition() == typeof(List<>))
{
List<T> listItems = (List<T>)items;
// 对整数数组进行升序排序
if (typeof(int).IsAssignableFrom(elementType))
listItems.Sort();
// 对字符串数组进行降序排序
else if (typeof(string).IsAssignableFrom(elementType))
listItems.Sort((x, y) => string.Compare(y, x, StringComparison.Ordinal)); // 字符降序
}
else
{
throw new ArgumentException("Unsupported data type for sorting");
}
// 输出排序后的结果
foreach (T item in items)
{
Console.WriteLine(item);
}
}
}
// 示例用法
class Program
{
static void Main()
{
int[] numbers = { 5, 2, 8, 1 };
string[] words = { "cat", "dog", "zebra" };
Sortable<int> numberSorter = new Sortable<int>();
numberSorter.Sort(numbers);
Sortable<string> wordSorter = new Sortable<string>();
wordSorter.Sort(words);
}
}
```
这个 `Sortable` 类接受一个泛型类型的数组作为输入,并根据元素的类型(`int` 或 `string`)进行相应的排序操作。注意,这里假设了输入的列表不是 `null`。
阅读全文
相关推荐
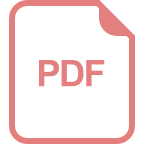
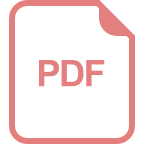
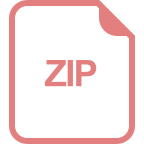
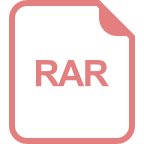
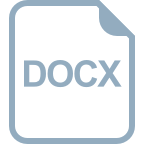
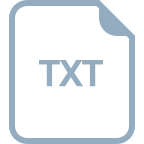
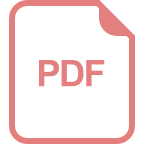
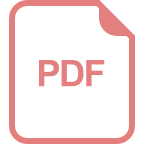
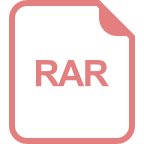
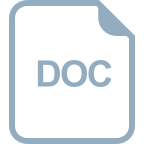
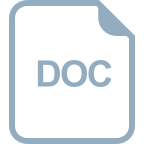
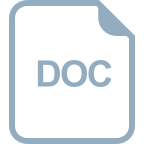
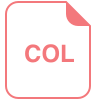
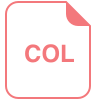




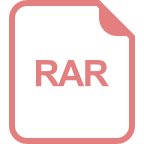