RuntimeError: only Tensors of floating point and complex dtype can require gradients :loss = loss_fn(top_indices, input_answer) loss.backward()
时间: 2024-04-13 17:28:40 浏览: 16
这个错误通常是因为您的损失函数`loss_fn`使用了不支持梯度计算的数据类型。在PyTorch中,只有浮点数和复数类型的张量才能够进行梯度计算。
要解决这个问题,您可以将损失函数的输入转换为浮点数类型。可以使用`.float()`方法将整数类型的张量转换为浮点数类型。请修改您的代码如下:
```python
import torch
# 假设top_indices为您通过torch.topk找到的最大值索引
# 将top_indices转换为浮点数类型
top_indices = top_indices.float()
# 计算损失函数
loss = loss_fn(top_indices, input_answer)
# 反向传播
loss.backward()
```
在上述代码中,我们使用`.float()`方法将`top_indices`转换为浮点数类型,以满足损失函数对数据类型的要求。然后,您可以继续计算损失值和进行反向传播。
希望这个解决方案对您有所帮助!如果还有其他问题,请随时提问。
相关问题
loss = loss_fn(top_indices.float(), input_answer.float()) loss.backward() RuntimeError: element 0 of tensors does not require grad and does not have a grad_fn
这个错误提示表明你正在尝试对一个不需要梯度计算的张量进行反向传播。在你的代码中,`top_indices`是通过解码器生成的预测结果,它并没有设置`requires_grad=True`,因此没有梯度函数。
为了解决这个问题,你可以考虑使用其他可训练的张量来计算损失,而不是直接使用`top_indices`。通常情况下,我们会使用模型的预测结果与真实标签之间的差异作为损失。
在你的代码中,可以尝试将`logits`作为计算损失的输入,而不是`top_indices`。然后,可以使用适当的损失函数(如交叉熵损失)计算模型的损失。示例如下:
```python
logits = self.decoder(context_vector)
loss = loss_fn(logits, input_answer)
loss.backward()
```
请确保在使用`loss.backward()`之前,所有需要梯度计算的张量都设置了`requires_grad=True`。如果仍然遇到问题,请检查其他部分的代码是否正确设置了梯度属性。
loss.backward()出现RuntimeError: element 0 of tensors does not require grad and does not have a grad_fn
这个错误通常是由于在计算图中存在不需要梯度计算的张量或变量导致的。
解决方法:
1.检查代码中的所有变量和张量,确保它们都设置了`requires_grad=True`,以便在反向传播时计算梯度。
2.如果确实存在不需要梯度计算的变量或张量,请在使用`backward()`函数之前将其从计算图中分离。可以使用`detach()`函数或`with torch.no_grad()`上下文管理器来实现。
下面是一个示例代码:
```
import torch
x = torch.tensor([1.0, 2.0, 3.0], requires_grad=True)
y = x * 2
z = y.mean()
# detach the variable from the computation graph
w = torch.tensor([2.0, 2.0, 2.0])
y_detached = y.detach()
# calculate gradients
z.backward()
x_grad = x.grad
# calculate gradients for detached variable
y_detached.backward(w)
x_grad_detached = x.grad
print(x_grad) # output: tensor([0.6667, 0.6667, 0.6667])
print(x_grad_detached) # output: tensor([2., 2., 2.])
```
在上面的例子中,我们通过将变量`y`分离到`y_detached`中来避免了计算图中的梯度问题。`y_detached`在计算图上与`y`的位置不同,因此不需要计算梯度。然后我们使用`backward()`函数计算`z`对`x`的梯度,以及使用`w`计算`y_detached`对`x`的梯度。最后,我们比较了这两个梯度的结果。
相关推荐
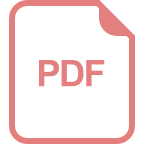
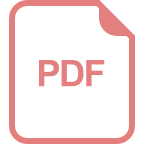
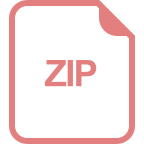









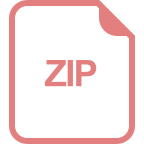
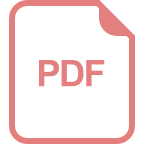
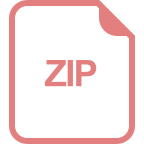