使用cv2.line绘制一个边长200个像素在等边三角形
时间: 2024-09-23 15:04:37 浏览: 175
在Python的OpenCV库`cv2`中,你可以通过以下几个步骤来绘制一个边长为200像素的等边三角形:
1. 导入必要的库:
```python
import cv2
import numpy as np
```
2. 设置图像尺寸和颜色模式:
```python
img_size = 400
image = np.zeros((img_size, img_size, 3), dtype=np.uint8)
```
这里我们创建一个400x400的全黑图像。
3. 定义三角形顶点坐标(假设中心点为(200, 200)):
```python
top_left = (50, 250)
bottom_left = (350, 50)
center = (200, 200)
```
4. 使用`cv2.line()`函数画出三条线:
```python
# 从中心到左上角
cv2.line(image, center, top_left, (255, 0, 0), thickness=200, lineType=cv2.LINE_AA)
# 从左下角到中心
cv2.line(image, bottom_left, center, (255, 0, 0), thickness=200, lineType=cv2.LINE_AA)
# 从中心到右上角
cv2.line(image, center, (150, 450), (255, 0, 0), thickness=200, lineType=cv2.LINE_AA)
```
这里我们将线条颜色设为红色(BGR),线宽设为200像素,确保了三角形的清晰。
完整的代码示例:
```python
import cv2
import numpy as np
img_size = 400
image = np.zeros((img_size, img_size, 3), dtype=np.uint8)
top_left = (50, 250)
bottom_left = (350, 50)
center = (200, 200)
# 绘制三角形
cv2.line(image, center, top_left, (255, 0, 0), thickness=200, lineType=cv2.LINE_AA)
cv2.line(image, bottom_left, center, (255, 0, 0), thickness=200, lineType=cv2.LINE_AA)
cv2.line(image, center, (150, 450), (255, 0, 0), thickness=200, lineType=cv2.LINE_AA)
# 显示结果
cv2.imshow("Triangular Line", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
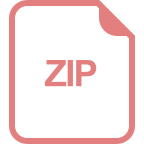
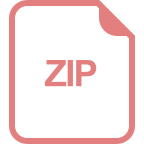
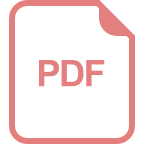

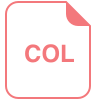
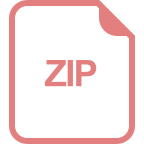
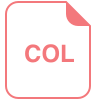









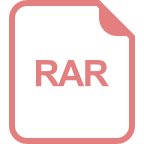