帮我用PyQt5设计一个设计一个800px*600px上左右结构的窗口,其中上面窗口是一个名为“top”的标题栏。标题栏内写入”基于大数据的股票预测系统“;左边为一个名为“left"股票选择的下拉框,可以选择对应的股票,股票选择下拉下拉框旁边为一个能够根据股票的选择显示对应的股票代码和一个提交按钮,其宽度为300px;右边为一个名为”right“的新闻列表和模型选择下拉框以及一个开始预测的提交按钮,其宽度为450px;
时间: 2024-03-12 08:42:59 浏览: 12
以下是一个符合您要求的PyQt5窗口设计代码:
```python
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QComboBox, QPushButton, QListWidget, QListWidgetItem, QVBoxLayout, QHBoxLayout
class StockPredictionSystem(QWidget):
def __init__(self):
super().__init__()
# 设置窗口大小
self.setGeometry(100, 100, 800, 600)
# 创建标题栏
title_label = QLabel('基于大数据的股票预测系统', self)
title_label.setObjectName('top')
# 创建股票选择下拉框
stock_combo_box = QComboBox(self)
stock_combo_box.setFixedWidth(200)
stock_combo_box.addItems(['股票A', '股票B', '股票C'])
# 创建显示股票代码的标签
stock_code_label = QLabel('股票代码:', self)
stock_code_label.setFixedWidth(100)
# 创建提交按钮
submit_button = QPushButton('提交', self)
submit_button.setFixedWidth(100)
# 创建左侧布局
left_layout = QHBoxLayout()
left_layout.addWidget(stock_combo_box)
left_layout.addWidget(stock_code_label)
left_layout.addWidget(submit_button)
left_layout.setContentsMargins(20, 20, 20, 20)
# 创建新闻列表
news_list = QListWidget(self)
news_list.setObjectName('right')
news_list.setFixedWidth(400)
news_list.addItem(QListWidgetItem('新闻A'))
news_list.addItem(QListWidgetItem('新闻B'))
news_list.addItem(QListWidgetItem('新闻C'))
# 创建模型选择下拉框
model_combo_box = QComboBox(self)
model_combo_box.setFixedWidth(200)
model_combo_box.addItems(['模型A', '模型B', '模型C'])
# 创建开始预测按钮
predict_button = QPushButton('开始预测', self)
predict_button.setFixedWidth(100)
# 创建右侧布局
right_layout = QVBoxLayout()
right_layout.addWidget(news_list)
right_layout.addWidget(model_combo_box)
right_layout.addWidget(predict_button)
right_layout.setContentsMargins(20, 20, 20, 20)
# 创建主布局
main_layout = QVBoxLayout()
main_layout.addWidget(title_label)
main_layout.addLayout(left_layout)
main_layout.addLayout(right_layout)
main_layout.setContentsMargins(0, 0, 0, 0)
# 设置主布局
self.setLayout(main_layout)
# 设置样式表
self.setStyleSheet('''
#top {
background-color: #007bff;
color: white;
font-size: 24px;
font-weight: bold;
padding: 20px;
}
#right {
background-color: #f2f2f2;
border: none;
font-size: 18px;
padding: 20px;
}
QListWidget::item {
border-bottom: 1px solid #ccc;
padding: 10px;
}
QListWidget::item:hover {
background-color: #e6e6e6;
}
QPushButton {
background-color: #007bff;
color: white;
border-radius: 5px;
border: none;
font-size: 18px;
font-weight: bold;
padding: 10px;
}
QPushButton:hover {
background-color: #0069d9;
}
QPushButton:pressed {
background-color: #0062cc;
}
''')
# 设置窗口标题
self.setWindowTitle('基于大数据的股票预测系统')
if __name__ == '__main__':
app = QApplication([])
window = StockPredictionSystem()
window.show()
app.exec_()
```
在上面的代码中,我们使用了QLabel、QComboBox、QPushButton和QListWidget等PyQt5组件来创建窗口中的各个元素。我们使用了QHBoxLayout和QVBoxLayout等PyQt5布局管理器来管理窗口中的各个元素的布局。同时,我们也使用了样式表来美化窗口中的各个元素的外观。
相关推荐
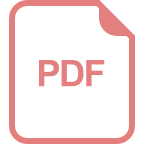














