10、需求:使用集合存储学生姓名、英语成绩、数学成绩、微积分成绩、总成绩从键盘录入以上数据,如:zhangshan,30,40,60,并计算出总成绩把学生信息和计算出来的总成绩存储到集合中,并按总成绩从低到高的顺序打印在控制台上
时间: 2023-07-04 22:23:18 浏览: 113
好的,这是一个关于集合的 Java 编程题目。下面是代码实现:
```java
import java.util.*;
public class Student {
String name;
int englishScore;
int mathScore;
int calculusScore;
int totalScore;
public Student(String name, int englishScore, int mathScore, int calculusScore) {
this.name = name;
this.englishScore = englishScore;
this.mathScore = mathScore;
this.calculusScore = calculusScore;
this.totalScore = englishScore + mathScore + calculusScore;
}
public String getName() {
return name;
}
public int getTotalScore() {
return totalScore;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
List<Student> studentList = new ArrayList<>();
System.out.println("请输入学生信息,格式为:姓名 英语成绩 数学成绩 微积分成绩");
while (scanner.hasNextLine()) {
String input = scanner.nextLine();
if (input.equals("exit")) {
break;
}
String[] inputs = input.split(" ");
String name = inputs[0];
int englishScore = Integer.parseInt(inputs[1]);
int mathScore = Integer.parseInt(inputs[2]);
int calculusScore = Integer.parseInt(inputs[3]);
Student student = new Student(name, englishScore, mathScore, calculusScore);
studentList.add(student);
}
Collections.sort(studentList, Comparator.comparingInt(Student::getTotalScore));
System.out.println("按总成绩从低到高的顺序打印学生信息:");
for (Student student : studentList) {
System.out.println(student.getName() + " " + student.getTotalScore());
}
}
}
```
上述代码实现了从键盘读取学生信息,将学生信息存储到集合中,并按总成绩从低到高的顺序打印学生信息。在实现过程中,我们定义了一个 `Student` 类来保存学生信息,并用 `List` 集合来存储学生对象。在读取学生信息时,我们使用了 `Scanner` 类来从键盘读取输入,并用 `split` 方法将其拆分成各个字段。最后,我们使用 `Collections.sort` 方法和 `Comparator` 接口来对学生信息进行排序。
阅读全文
相关推荐










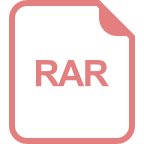

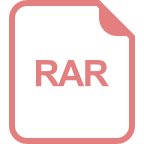





