请帮我使用jasvScript向上修改树形结构数据每个父节点中指定的字段属性值
时间: 2023-11-22 10:53:57 浏览: 62
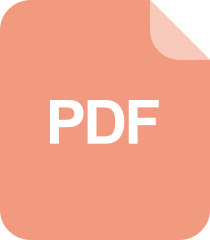
php实现的树形结构数据存取类实例
可以使用递归来遍历树形结构数据,并在每个父节点中修改指定的字段属性值。以下是一个示例代码:
```javascript
function updateParentNodes(treeData, fieldName, fieldValue) {
if (!treeData || treeData.length === 0) {
return;
}
for (let i = 0; i < treeData.length; i++) {
const node = treeData[i];
if (node.children && node.children.length > 0) {
// 递归遍历子节点
updateParentNodes(node.children, fieldName, fieldValue);
}
if (node.parentId) {
// 修改父节点的指定字段属性值
const parentNode = findNodeById(treeData, node.parentId);
if (parentNode) {
parentNode[fieldName] = fieldValue;
}
}
}
}
// 根据节点id查找节点
function findNodeById(treeData, nodeId) {
if (!treeData || treeData.length === 0) {
return null;
}
for (let i = 0; i < treeData.length; i++) {
const node = treeData[i];
if (node.id === nodeId) {
return node;
}
if (node.children && node.children.length > 0) {
const result = findNodeById(node.children, nodeId);
if (result) {
return result;
}
}
}
return null;
}
```
使用示例:
```javascript
const treeData = [
{
id: 1,
name: 'Parent 1',
parentId: null,
count: 0,
children: [
{
id: 2,
name: 'Child 1',
parentId: 1,
count: 0,
children: [
{
id: 3,
name: 'Grandchild 1',
parentId: 2,
count: 0,
children: []
}
]
}
]
},
{
id: 4,
name: 'Parent 2',
parentId: null,
count: 0,
children: []
}
];
updateParentNodes(treeData, 'count', 1);
console.log(treeData);
```
输出结果:
```javascript
[
{
id: 1,
name: 'Parent 1',
parentId: null,
count: 1,
children: [
{
id: 2,
name: 'Child 1',
parentId: 1,
count: 1,
children: [
{
id: 3,
name: 'Grandchild 1',
parentId: 2,
count: 1,
children: []
}
]
}
]
},
{
id: 4,
name: 'Parent 2',
parentId: null,
count: 1,
children: []
}
]
```
可以看到,每个父节点中的 `count` 属性值都被修改为了 1。
阅读全文
相关推荐
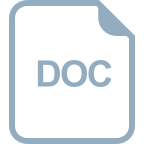
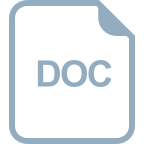



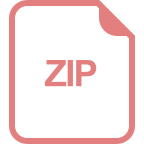
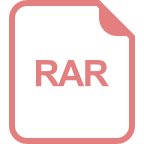
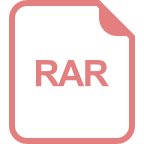
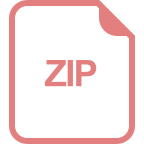
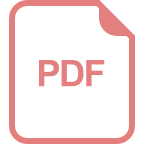
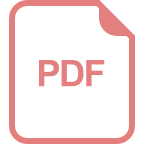
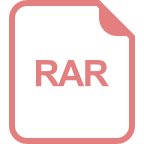
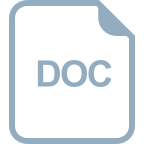
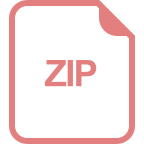
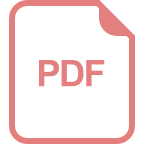
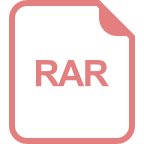
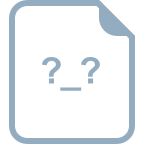
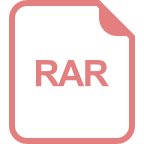