我的意思是根据findContours函数之后输出的contours来在ui上绘制轮廓,并用鼠标对这些轮廓进行编辑
时间: 2024-04-07 08:28:18 浏览: 114
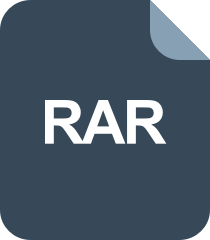
鼠标捕捉轮廓
你可以使用OpenCV中的drawContours函数来在UI上绘制轮廓。具体步骤如下:
1. 使用findContours函数获取轮廓信息,并将其存储在一个vector中。
2. 在UI上创建一个画布,并将图片显示在上面。
3. 使用drawContours函数将轮廓绘制在画布上。
4. 添加鼠标事件监听器,在鼠标点击轮廓时,根据鼠标位置编辑轮廓。
以下是一个简单的示例代码:
```c++
#include <opencv2/opencv.hpp>
using namespace cv;
// 鼠标事件回调函数
void onMouse(int event, int x, int y, int flags, void* param)
{
if (event == EVENT_LBUTTONDOWN)
{
// 在此处处理鼠标点击事件
}
}
int main()
{
// 读取图片
Mat img = imread("test.jpg", IMREAD_GRAYSCALE);
// 获取轮廓信息
std::vector<std::vector<Point>> contours;
std::vector<Vec4i> hierarchy;
findContours(img, contours, hierarchy, RETR_TREE, CHAIN_APPROX_SIMPLE);
// 创建画布并显示图片
Mat canvas = Mat::zeros(img.size(), CV_8UC3);
cvtColor(img, canvas, COLOR_GRAY2BGR);
imshow("Canvas", canvas);
// 绘制轮廓
drawContours(canvas, contours, -1, Scalar(0, 0, 255), 2);
imshow("Canvas", canvas);
// 添加鼠标事件监听器
setMouseCallback("Canvas", onMouse, NULL);
waitKey(0);
return 0;
}
```
在onMouse函数中,你可以根据鼠标位置和轮廓信息,实现对轮廓的编辑。例如,你可以使用pointPolygonTest函数来判断鼠标位置是否在轮廓内部,然后根据需要对轮廓进行修改。
阅读全文
相关推荐
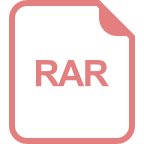
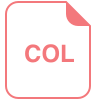



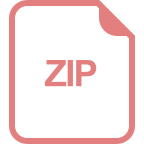
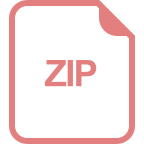
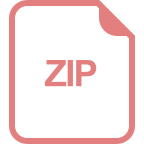
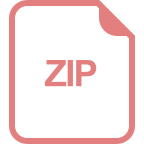
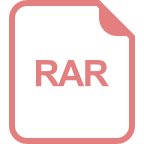
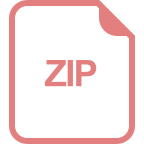