4.编写一个程序实现类似DOS命令copy的文件拷贝功能(教材练习题6)。要求用两种方法实现:(1)拷贝的源文件和目标文件由命令行输入;(2)拷贝的源文件和目标文件按对话方式输入。
时间: 2024-03-16 12:44:51 浏览: 21
以下是实现文件拷贝功能的程序,包括两种方法:
方法一:通过命令行输入文件名
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main(int argc, char* argv[]) {
if (argc != 3) {
cout << "Usage: copy <source_file> <target_file>" << endl;
return 1;
}
string source_file = argv[1];
string target_file = argv[2];
ifstream source(source_file.c_str(), ios::binary);
if (!source) {
cout << "Failed to open source file: " << source_file << endl;
return 1;
}
ofstream target(target_file.c_str(), ios::binary);
if (!target) {
cout << "Failed to open target file: " << target_file << endl;
return 1;
}
target << source.rdbuf();
source.close();
target.close();
cout << "Copy completed." << endl;
return 0;
}
```
方法二:通过对话方式输入文件名
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
string source_file, target_file;
// 读取源文件名
cout << "Please enter source file name: ";
getline(cin, source_file);
// 读取目标文件名
cout << "Please enter target file name: ";
getline(cin, target_file);
ifstream source(source_file.c_str(), ios::binary);
if (!source) {
cout << "Failed to open source file: " << source_file << endl;
return 1;
}
ofstream target(target_file.c_str(), ios::binary);
if (!target) {
cout << "Failed to open target file: " << target_file << endl;
return 1;
}
target << source.rdbuf();
source.close();
target.close();
cout << "Copy completed." << endl;
return 0;
}
```
这两个程序的工作方式相同,都是将一个文件复制到另一个文件。第一个程序通过命令行输入文件名,第二个程序通过对话方式读取文件名。这两种方法都使用了C++中的文件流。打开文件时,使用了ios::binary模式,以确保文件以二进制模式打开,而不是文本模式。最后,将源文件的内容写入目标文件中,并关闭文件流。
相关推荐
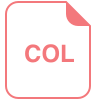
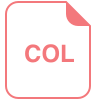
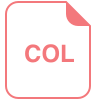
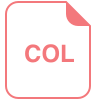
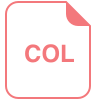









