用c语言写一段完整的代码 实现单循环链表的初始化、求数据元素个数、插入、删除、取数据元素等操作; 用插入法建立带头结点的单循环链表;要求把单链表以头文件形式保存且采用模块化程序设计方法实现单循环链表。
时间: 2024-10-08 21:11:33 浏览: 39
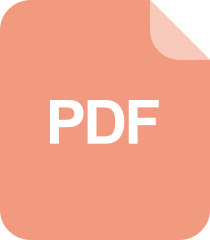
C语言单循环链表的表示与实现实例详解
以下是使用 C 语言实现单循环链表的一些基本操作的代码示例。我们将创建一个名为`single_linked_list.h`的头文件,以及一个名为`single_linked_list.c`的实现文件。
**single_linked_list.h**
```c
#ifndef SINGLE_LINKED_LIST_H
#define SINGLE_LINKED_LIST_H
// 定义链表节点结构体
typedef struct Node {
int data; // 节点的数据
struct Node* next; // 指向下一个节点的指针
} Node;
// 单循环链表结构体
typedef struct SingleLinkedList {
Node* head; // 链表头部
int length; // 链表长度
} SingleLinkedList;
// 初始化链表
SingleLinkedList* create_list();
// 插入节点
void insert_node(SingleLinkedList* list, int value, int position);
// 删除节点
void delete_node(SingleLinkedList* list, int index);
// 取数据元素
int get_data_element(const SingleLinkedList* list, int index);
// 获取链表元素个数
int get_length(const SingleLinkedList* list);
#endif // SINGLE_LINKED_LIST_H
```
**single_linked_list.c**
```c
#include "single_linked_list.h"
// 实现函数
SingleLinkedList* create_list() {
SingleLinkedList* list = (SingleLinkedList*)malloc(sizeof(SingleLinkedList));
list->head = NULL;
list->length = 0;
return list;
}
void insert_node(SingleLinkedList* list, int value, int position) {
if (position <= 0 || position > list->length + 1) {
printf("Invalid position.\n");
return;
}
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = list->head;
if (position == 1) {
newNode->next->next = newNode;
} else {
Node* current = list->head;
for (int i = 1; i < position - 1; i++) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
list->length++;
}
void delete_node(SingleLinkedList* list, int index) {
if (index < 1 || index > list->length) {
printf("Invalid index.\n");
return;
}
if (index == 1) {
Node* temp = list->head;
list->head = list->head->next;
free(temp);
} else {
Node* current = list->head;
for (int i = 1; i < index - 1; i++) {
current = current->next;
}
Node* temp = current->next;
current->next = temp->next;
free(temp);
}
list->length--;
}
int get_data_element(const SingleLinkedList* list, int index) {
if (index < 1 || index > list-1; // 或者抛出异常
}
Node* current = list->head;
for (int i = 1; i < index; i++) {
current = current->next;
}
return current->data;
}
int get_length(const SingleLinkedList* list) {
return list->length;
}
```
使用这个代码,你可以分别导入`single_linked_list.h`并实现链表的初始化、插入、删除、取值和获取长度的操作。注意,这里没有处理所有边界条件,实际项目中需要完善错误检查和处理。
阅读全文
相关推荐
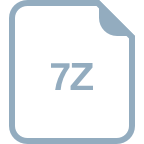
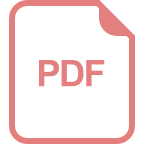









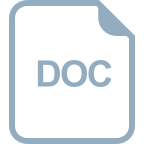
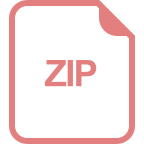
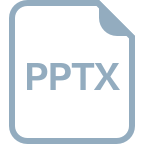
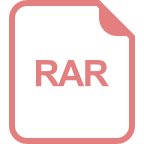
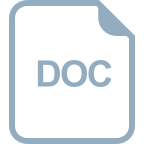
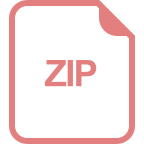
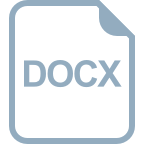
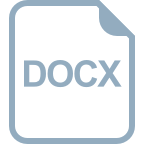