JAVA中使用springboot集成axis发布webservice服务端 示例
时间: 2023-07-29 11:14:38 浏览: 72
以下是使用Spring Boot集成Apache Axis2发布Web Service服务端的示例:
1. 首先,需要在pom.xml中添加Axis2和Spring Boot的依赖:
```xml
<dependency>
<groupId>org.apache.axis2</groupId>
<artifactId>axis2-webapp</artifactId>
<version>1.7.9</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
2. 创建一个简单的Web Service服务端:
```java
package com.example.demo;
import org.apache.axis2.context.MessageContext;
import org.apache.axis2.transport.http.HTTPConstants;
import org.springframework.stereotype.Component;
@Component
public class MyService {
public String sayHello() {
MessageContext messageContext = MessageContext.getCurrentMessageContext();
String userAgent = (String) messageContext.getProperty(HTTPConstants.HEADER_USER_AGENT);
return "Hello, " + userAgent + "!";
}
}
```
3. 创建一个发布Web Service服务端的配置类:
```java
package com.example.demo;
import org.apache.axis2.AxisFault;
import org.apache.axis2.context.ConfigurationContext;
import org.apache.axis2.context.ConfigurationContextFactory;
import org.apache.axis2.description.AxisService;
import org.apache.axis2.engine.AxisConfiguration;
import org.apache.axis2.transport.http.server.AxisHttpService;
import org.apache.axis2.transport.http.server.HttpServiceHandler;
import org.apache.axis2.transport.http.server.SimpleHttpServer;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import javax.annotation.PostConstruct;
import javax.annotation.PreDestroy;
import java.net.InetSocketAddress;
@Configuration
public class WebServiceConfiguration {
private SimpleHttpServer server;
@Autowired
private MyService myService;
@PostConstruct
public void start() throws Exception {
ConfigurationContext configurationContext = ConfigurationContextFactory.createDefaultConfigurationContext();
AxisConfiguration axisConfiguration = configurationContext.getAxisConfiguration();
AxisService axisService = new AxisService("MyService");
axisService.addParameter("ServiceClass", MyService.class.getName());
axisService.addMethod("sayHello");
axisConfiguration.addService(axisService);
HttpServiceHandler handler = new HttpServiceHandler(configurationContext);
AxisHttpService axisHttpService = new AxisHttpService(axisConfiguration, handler);
server = new SimpleHttpServer();
server.setBindAddress(new InetSocketAddress(8080));
server.deploy(axisHttpService);
server.start();
}
@PreDestroy
public void stop() throws AxisFault {
server.stop();
}
@Bean
public MyService myService() {
return new MyService();
}
}
```
4. 运行Spring Boot应用程序并访问http://localhost:8080/axis2/services/MyService?wsdl,应该能够看到服务的WSDL描述。
现在,您已经成功地使用Spring Boot集成Apache Axis2发布Web Service服务端。
相关推荐
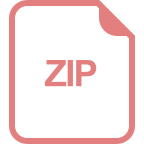














