代码实现链式队列,输入数字入队,输入字符出队。c语言
时间: 2024-01-15 12:09:38 浏览: 79
以下是基于单链表实现的链式队列的代码示例,实现了输入数字入队,输入字符出队的功能:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node;
typedef struct queue {
Node *front; // 队头指针
Node *rear; // 队尾指针
} Queue;
// 初始化队列
void initQueue(Queue *q) {
q->front = q->rear = (Node*) malloc(sizeof(Node));
q->front->next = NULL;
}
// 入队
void enQueue(Queue *q, int data) {
Node *newNode = (Node*) malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
q->rear->next = newNode;
q->rear = newNode;
}
// 判空
int isEmpty(Queue *q) {
return q->front == q->rear;
}
// 出队
int deQueue(Queue *q) {
if (isEmpty(q)) {
printf("队列为空!\n");
return -1;
}
Node *p = q->front->next;
int data = p->data;
q->front->next = p->next;
if (q->rear == p) q->rear = q->front;
free(p);
return data;
}
// 测试
int main() {
Queue q;
initQueue(&q);
char c;
while(1) {
scanf("%c", &c);
if (c >= '0' && c <= '9') {
int num = c - '0';
enQueue(&q, num);
} else if (c == '\n') {
continue;
} else {
int data = deQueue(&q);
if (data != -1) printf("%d ", data);
}
}
return 0;
}
```
以上代码实现了数字入队,字符出队的功能,其中利用了队列的先进先出特性,通过将数字依次入队,再按照输入字符进行出队操作,实现了该功能。
阅读全文
相关推荐



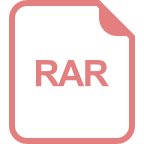
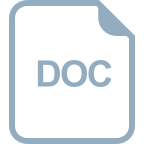
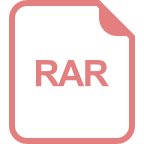
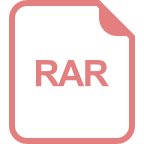


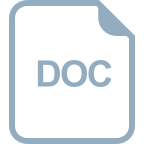






